Login registration with reset password screen using flutter | Login | Registration | Forget password | flutter | iOS | Android,
First, there is a widget for the organization/association/application name. Then about the actual screen, Sign in. Presently, we have two text fields, email and password key, to get login/sign-in credentials from a user from the client.
Then we have a used widget for the link to Forgot password and navigation of the screen, and we used the background screen for the normal size of the layout.

Environment
- 1.71.0 (Universal)
- Dart Programming
- Flutter 3.0.5+ Version
- Build iOS
- Build Android
- Run iPhone 11 Pro
- Android SDK
- XCODE
- CMD (on Windows) or Terminal Terminal (on Mac/Linux)
- IDE (Android Studio/IntelliJ/Visual Studio Code)
Features structure
- MVC structure
- Refactor data model and userDefault
- A lot of enum used
- Delegate pass value
- ListView UI Design
- Widgets
- Button
Pods(Pub.dev)
- path_provider: ^2.0.8
- shared_preferences: ^2.0.7
- fluttertoast: ^8.0.8
- flutter_spinkit: ^5.1.0
- intl_utils: ^2.7.0
- package_info: ^2.0.0
- permission_handler: ^6.0.1+1
- google_fonts: ^2.1.0
- progress_dialog_null_safe: ^1.0.6
Flutter Intro
Flutter transforms the app development process. Build, test, and deploy beautiful mobile, web, desktop, and embedded apps from a single codebase. Flutter is an open-source framework by Google for building beautiful, natively compiled, multi-platform applications from a single codebase (Flutter References)
Login in flutter using API Use Layout (Screens)
Login:- The login screen most important used scenario of any mobile app and website page or an entry page to a web/mobile application that requires user identification and authentication, regularly performed by entering a username and password combination as per the required combination.
Registration screen:- We have provided the features of our app used for the common person to use the full app for required registration for any app is a major screen to enter the correct information and fill up some basic requirement full file by the users after that used the app resolved the user problem.
Forget password:-This screen is play a major role in any app for identification and authentication to use the services for the existing app. users change the password according to our requirements and re-login after creating a new password.
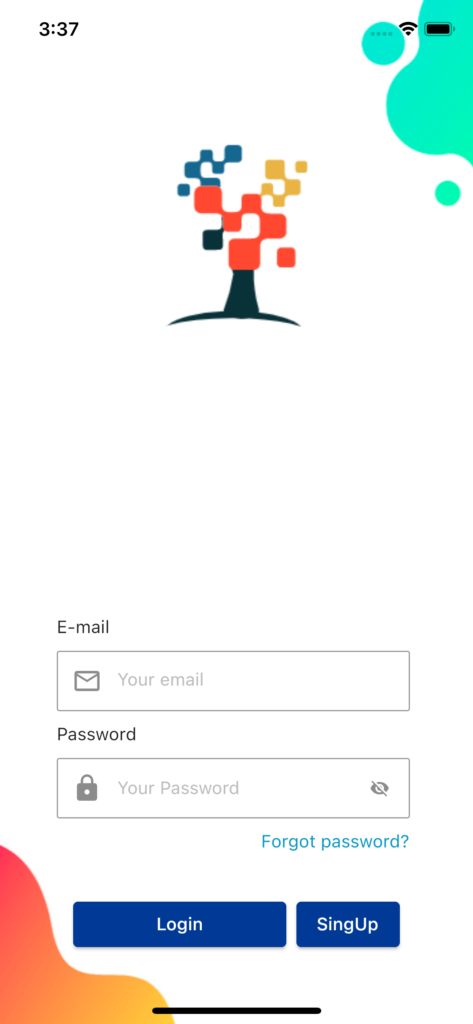
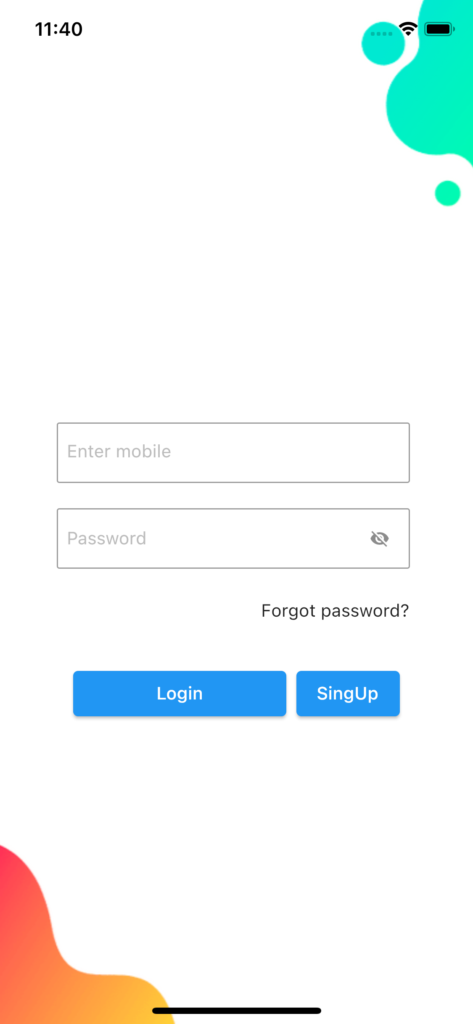
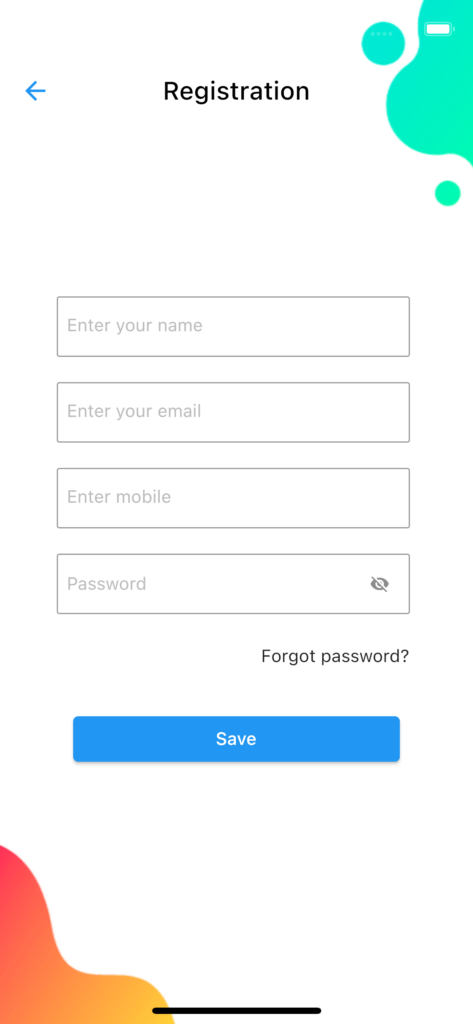
Output Screen:- Login with custom UI and Registration layout.
Let’s get started on the main steps!
Main Screen
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:my_app/l10n/l10n.dart';
import '<a href="package:my_app">package:my_app</a>/language_setup/local_language.dart';
import 'package:my_app/mainapp/main_login.dart';
import 'package:flutter_localizations/flutter_localizations.dart';
import 'package:flutter_gen/gen_l10n/app_localizations.dart';
import 'package:get/get.dart';
import 'package:my_app/mainapp/password/password_success_screen.dart';
import 'package:my_app/mainapp/policy_registration.dart';
import 'package:my_app/mainapp/password/secu_eset_password.dart';
import 'package:my_app/mainapp/singup_form.dart';
import 'package:my_app/other_desgin/screens/google_map.dart';
import 'package:my_app/other_desgin/screens/home/main_screen.dart';
import 'package:my_app/other_desgin/screens/login_and_reg/login.dart';
// import 'package:flutter_native_splash/flutter_native_splash.dart';
import 'package:my_app/utlity/colors.dart';
import 'package:lottie/lottie.dart';
void main() {
WidgetsFlutterBinding.ensureInitialized();
// FlutterNativeSplash.preserve(widgetsBinding: widgetsBinding);
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: '',
debugShowCheckedModeBanner: false,
theme: ThemeData(
// primarySwatch: Colors.blue,
// platform: TargetPlatform.iOS,
),
translations: LocaleString(),
locale: const Locale('en', 'US'),
// locale: provider.locale,
supportedLocales: L10n.all,
// ignore: prefer_const_literals_to_create_immutables
localizationsDelegates: [
// RefreshLocalizations.delegate,
AppLocalizations.delegate,
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
GlobalCupertinoLocalizations.delegate,
],
home: loginMain(),
routes: {
"/mainlogin": (context) => MainLogin(),
"/singUp": (context) => SignupForm(),
"/selectLocation": (context) => GoogleMapForm(),
"/dashboard": (context) => DashboardForm(),
"/policyReg": (context) => PolicyRegistration(),
"/loginMain": (context) => MainLogin(),
"/ResetPassword": (context) => ResetPassword(),
"/successScreen": (context) => SuccessScreen(),
});
}
}
main screen with connecting the login screen after the splash screen
Login Screen
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
import 'package:my_app/other_desgin/screens/choose_language.dart';
import 'package:my_app/other_desgin/screens/google_map.dart';
import 'package:my_app/other_desgin/screens/login_and_reg/forget_pass.dart';
import 'package:my_app/other_desgin/screens/reg_form.dart';
import 'package:my_app/utlity/colors.dart';
import 'package:my_app/widgets/bg_image.dart';
class loginMain extends StatefulWidget {
@override
State<loginMain> createState() => _loginMainState();
}
class _loginMainState extends State<loginMain> {
final emailController = TextEditingController();
final passController = TextEditingController();
bool isShowPass = false;
bool onError = false;
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/images/logoin.png"),
fit: BoxFit.cover,
),
),
child: Scaffold(
// appBar: AppBar(
// title: Text(''),
// ),
backgroundColor: Colors.transparent, //AppColors.mainBg, //
body: Container(
padding: EdgeInsets.symmetric(horizontal: 15, vertical: 10),
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
BgImage(),
// Padding(
// padding: const EdgeInsets.only(left: 24, right: 20),
// child: Image.asset(
// "assets/images/subLogo.png",
// fit: BoxFit.cover,
// ),
// ),
SizedBox(
height: 15,
),
SizedBox(
height: 200,
),
Padding(
padding: const EdgeInsets.only(left: 30.0),
child: Text('E-mail'),
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
style: TextStyle(color: Colors.black, fontSize: 14),
controller: emailController,
decoration: InputDecoration(
prefixIcon: Icon(Icons.email_outlined),
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
labelText: "Your email",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle:
TextStyle(color: Colors.grey.shade400, fontSize: 14),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = new RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child: Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 30.0),
child: Text('Password'),
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
controller: passController,
obscureText: isShowPass,
decoration: InputDecoration(
prefixIcon: Icon(Icons.lock),
labelText: "Your Password",
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle:
TextStyle(color: Colors.grey.shade400, fontSize: 14),
suffixIcon: GestureDetector(
onTap: () {
setState(() {
isShowPass = !isShowPass;
});
},
child: Icon(
isShowPass
? Icons.visibility
: Icons.visibility_off,
size: 16,
)),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = new RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child: Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 10,
),
GestureDetector(
onTap: () => Navigator.push(context,
MaterialPageRoute(builder: (context) => ForgetPassword())),
child: Align(
alignment: Alignment.topRight,
child: Padding(
padding: const EdgeInsets.only(right: 35.0),
child: Text(
"Forgot password?",
style: TextStyle(color: AppColors.blueColor),
),
),
),
),
SizedBox(
height: 25,
),
Padding(
padding: EdgeInsets.only(left: 35, right: 35),
child: Container(
padding: EdgeInsets.all(8.0),
// height: 100,
child: Row(
children: [
Expanded(
child: Container(
//height: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppColors.buttonColor,
onPrimary: Colors.white,
side: BorderSide(
color: AppColors.buttonColor, width: 5),
),
onPressed: () async {
Navigator.pushAndRemoveUntil(
context,
MaterialPageRoute(
builder: (context) => ChooseLanguage()),
ModalRoute.withName("/chooseLaguage"));
},
child: Text("Login"),
),
)),
Container(
padding: EdgeInsets.only(left: 8.0),
//height: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppColors.buttonColor,
onPrimary: Colors.white,
side: BorderSide(
color: AppColors.buttonColor, width: 5),
),
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => regForm()));
},
child: Text("SingUp")))
],
),
),
),
Spacer(),
SizedBox(
height: 10,
),
],
),
),
),
);
}
}
the login screen adds the 2 texts filed one is a user name and 2nd is the password with an eye show and hide password enter the user and see the entered password.
Registration Screen
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
import 'package:my_app/other_desgin/screens/google_map.dart';
import 'package:my_app/other_desgin/screens/login_and_reg/google_map2.dart';
import 'package:my_app/other_desgin/screens/login_and_reg/map.dart';
import 'package:geocoding/geocoding.dart';
import 'package:geolocator/geolocator.dart';
import 'package:my_app/utlity/colors.dart';
import 'package:my_app/widgets/bg_image.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';
import 'package:intl_phone_field/intl_phone_field.dart';
class regForm extends StatefulWidget {
@override
State<regForm> createState() => _regFormState();
}
class _regFormState extends State<regForm> {
final _formKey = GlobalKey<FormState>();
final nameController = TextEditingController();
final mobileController = TextEditingController();
final emailController = TextEditingController();
final confirmPassController = TextEditingController();
final passController = TextEditingController();
String googleApikey = "your key";
GoogleMapController? mapController; //contrller for Google map
CameraPosition? cameraPosition;
LatLng startLocation = LatLng(26.4839, 80.27508);
String location = "Location Name:";
bool isShowPass = false;
bool onError = false;
Widget googleMapUI() {
double width = MediaQuery.of(context).size.width;
return SizedBox(
// height: MediaQuery.of(context).size.height / 1.8,
height: MediaQuery.of(context).size.height / 3,
width: width,
child: Stack(
children: <Widget>[
Padding(
padding: const EdgeInsets.only(top: 30),
child: Stack(children: [
GoogleMap(
//Map widget from google_maps_flutter package
zoomGesturesEnabled: true,
myLocationEnabled: true,
//enable Zoom in, out on map
initialCameraPosition: CameraPosition(
//innital position in map
target: startLocation, //initial position
zoom: 14.0, //initial zoom level
),
mapType: MapType.normal, //map type
onMapCreated: (controller) {
//method called when map is created
setState(() {
mapController = controller;
});
},
onCameraMove: (CameraPosition cameraPositiona) {
cameraPosition = cameraPositiona; //when map is dragging
},
onCameraIdle: () async {
//when map drag stops
List<Placemark> placemarks = await placemarkFromCoordinates(
cameraPosition!.target.latitude,
cameraPosition!.target.longitude);
setState(() {
//get place name from lat and lang
location = placemarks.first.street.toString() +
', ' +
placemarks.first.subAdministrativeArea.toString() +
',' +
placemarks.first.locality.toString() +
'-' +
placemarks.first.postalCode.toString() +
',' +
placemarks.first.administrativeArea.toString();
});
},
),
Center(
//picker image on google map
child: Image.asset(
"assets/images/picker.png",
width: 80,
),
),
])),
],
),
);
}
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/images/regBg.png"),
fit: BoxFit.cover,
),
),
child: Scaffold(
extendBodyBehindAppBar: true,
appBar: AppBar(
title: Text('Sign-up', style: TextStyle(color: Colors.black)),
backgroundColor: Colors.transparent,
leading: IconButton(
icon: Icon(Icons.arrow_back, color: Colors.red),
onPressed: () => Navigator.of(context).pop(),
),
elevation: 0,
),
backgroundColor: Colors.transparent,
body: Center(
child: SingleChildScrollView(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
googleMapUI(),
Container(
height: 2,
width: double.infinity,
color: Colors.amber,
),
Container(
height: 90,
child: Padding(
padding: EdgeInsets.only(left: 27, right: 29, bottom: 5),
child: Card(
child: Container(
padding: EdgeInsets.all(0),
width: MediaQuery.of(context).size.width - 40,
child: ListTile(
leading: Image.asset(
"assets/images/picker.png",
width: 25,
),
title: Text(
location,
style: TextStyle(fontSize: 18),
),
dense: true,
)),
),
),
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 4),
child: TextFormField(
style: TextStyle(color: Colors.black, fontSize: 14),
controller: emailController,
decoration: InputDecoration(
prefixIcon: Icon(Icons.account_circle_sharp),
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
labelText: "Enter your name",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle: TextStyle(
color: Colors.grey.shade400, fontSize: 14),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = new RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child:
Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 5,
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
style: TextStyle(color: Colors.black, fontSize: 14),
controller: emailController,
decoration: InputDecoration(
prefixIcon: Icon(Icons.email_rounded),
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
labelText: "Enter your email",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle: TextStyle(
color: Colors.grey.shade400, fontSize: 14),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = new RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child:
Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 5,
),
SizedBox(
height: 5,
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: Column(children: <Widget>[
Container(
child: IntlPhoneField(
decoration: InputDecoration(
//decoration for Input Field
labelText: 'Phone Number',
border: OutlineInputBorder(
borderSide: BorderSide(),
),
),
initialCountryCode:
'IN', //default contry code, NP for Nepal
onChanged: (phone) {
//when phone number country code is changed
print(phone.completeNumber); //get complete number
print(phone.countryCode); // get country code only
print(phone.number); // only phone number
},
)),
])),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child:
Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 5,
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
controller: nameController,
obscureText: isShowPass,
decoration: InputDecoration(
labelText: "Password",
alignLabelWithHint: true,
prefixIcon: Icon(Icons.lock),
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle: TextStyle(
color: Colors.grey.shade400, fontSize: 14),
suffixIcon: GestureDetector(
onTap: () {
setState(() {
isShowPass = !isShowPass;
});
},
child: Icon(
isShowPass
? Icons.visibility
: Icons.visibility_off,
size: 16,
)),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = new RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child:
Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 5,
),
Text('By signing up you agree to our'),
Text('and'),
Text('By signing up you agree to our'),
Padding(
padding: EdgeInsets.only(left: 35, right: 35),
child: Container(
padding: EdgeInsets.all(8.0),
// height: 100,
child: Row(
children: [
Expanded(
child: Container(
//height: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppColors.buttonColor,
onPrimary: Colors.white,
side: BorderSide(
color: AppColors.buttonColor, width: 5),
),
onPressed: () async {
},
child: Text("Next"),
),
],
),
),
),
],
),
),
)),
);
}
}
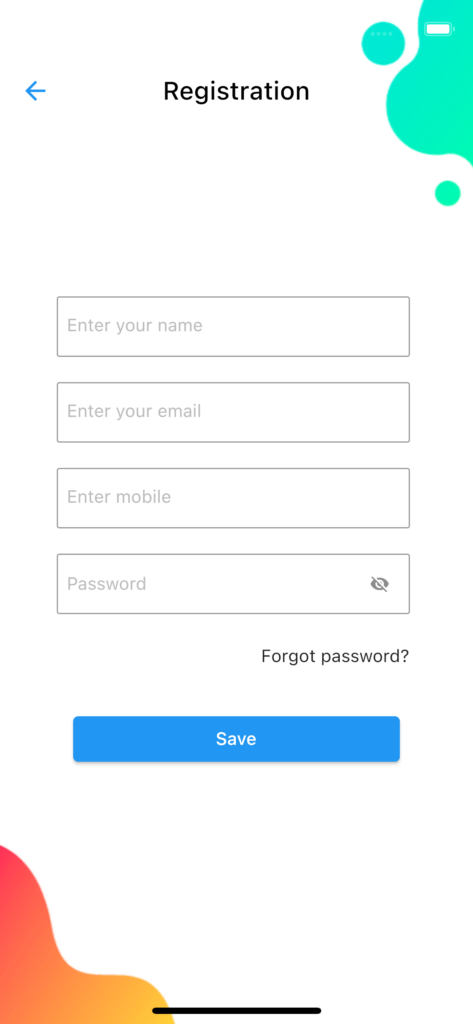
Forget Password
// ignore_for_file: prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
import 'package:my_app/other_desgin/screens/google_map.dart';
import 'package:my_app/other_desgin/screens/login_and_reg/google_map2.dart';
import 'package:my_app/other_desgin/screens/login_and_reg/map.dart';
import 'package:my_app/other_desgin/screens/reg_form.dart';
import 'package:my_app/utlity/colors.dart';
import 'package:my_app/widgets/bg_image.dart';
class ForgetPassword extends StatefulWidget {
@override
State<ForgetPassword> createState() => _ForgetPasswordState();
}
class _ForgetPasswordState extends State<ForgetPassword> {
final emailController = TextEditingController();
//final passController = TextEditingController();
bool isShowPass = false;
bool onError = false;
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/images/regBg.png"),
fit: BoxFit.cover,
),
),
child: Scaffold(
extendBodyBehindAppBar: true,
appBar: AppBar(
backgroundColor: AppColors.headerColor,
title: Text('', style: TextStyle(color: Colors.black)),
leading: IconButton(
// ignore: prefer_const_constructors
icon: Icon(Icons.arrow_back, color: Colors.red),
onPressed: () => Navigator.of(context).pop(),
),
elevation: 0,
),
backgroundColor: Colors.transparent,
body: Center(
child: SingleChildScrollView(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ForgetPasswordImage(),
// Container(
// height: 2,
// width: double.infinity,
// color: Colors.amber,
// ),
SizedBox(
height: 250,
),
Padding(
padding: EdgeInsets.only(left: 1, right: 230),
child: Text("@ Mobile Number"),
//"Don't worry! it happens.Please enter the \n address associated with your account."),
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
style: TextStyle(color: Colors.black, fontSize: 14),
controller: emailController,
decoration: InputDecoration(
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
labelText: "Enter mobile",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle: TextStyle(
color: Colors.grey.shade400, fontSize: 14),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child:
Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 5,
),
SizedBox(
height: 5,
),
Padding(
padding: EdgeInsets.only(left: 35, right: 35),
child: Container(
padding: EdgeInsets.all(8.0),
// height: 100,
child: Row(
children: [
Expanded(
child: Container(
//height: 100,
child: ElevatedButton(
onPressed: () async {
},
child: Text("Next"),
),
)),
],
),
),
),
],
),
),
)),
);
}
}
Color Class
import 'package:flutter/material.dart';
class AppColors {
static final Color spleshColor = Color(0XFFDEDEDE);
static final Color blueColor = Color(0XFF0c99c3);
static final Color greenColor = Color(0XFF3dc39d);
static final Color yellowColor = Color(0XFFdac007);
static final Color redColor = Color(0XFFbe0b2b);
static final Color orangeColor = Color(0XFFbe740b);
static const buttonColor = Color(0xFF003A96); //Color(0xFFF69BD44);
static const mainBg = Color(0xFFFAFEFE);
static const textRegColor = Color(0xFF1D4380);
static const textWaterColor = Color(0xFF747980);
static const headerColor = Color(0xFF1D4380); //new color Color(0xFFFCFCFC);
static const darkColor = Color(0xFF2A0B35);
static const grayColor = Color(0xFF767676);
static const listRowColor = Color(0xFFF0FFE9);
static const listRowcheckBoxColor = Color(0xFFFFFFFF);
static const searchLocationMapColor = Color(0xFFFAFFFE);
static const card1 = Color(0xFF2A694B);
static const card2 = Color(0xFF713838);
static const card3 = Color(0xFF3F4B69);
static const card4 = Color(0xFF696438);
static const card5 = Color(0xFF71416F);
static const card6 = Color(0xFF357D63);
static const cardPolicy = Color(0xFFEDFFE2);
static const cardParam = Color(0xFF578F9B);
static const textPolicyBlackColor = Color(0xFF2A0B35);
static const cardText = Color(0xFF1D4380);
static const policyCopenColor = Color(0xFFFCFCFC);
static const policyPaytColor = Color(0xFF767676);
static const cardColor = Color(0xFF1D4380);
static const loginScreenColor = Color(0xFF545454);
}
const mainBgColor = Color(0xFFf2f2f2);
const darkColor = Color(0xFF2A0B35);
const midColor = Color(0xFF522349);
const lightColor = Color(0xFFA52C4D);
const darkRedColor = Color(0xFFFA695C);
const lightRedColor = Color(0xFFFD685A);
const purpleGradient = LinearGradient(
colors: <Color>[darkColor, midColor, lightColor],
stops: [0.0, 0.5, 1.0],
begin: Alignment.centerLeft,
end: Alignment.centerRight,
);
const redGradient = LinearGradient(
colors: <Color>[darkRedColor, lightRedColor],
stops: [0.0, 1.0],
begin: Alignment.centerLeft,
end: Alignment.centerRight,
);
Image Class
import 'package:flutter/material.dart';
class BgImage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.only(top: 100.0, left: 24, right: 20),
child: Image.asset(
"assets/images/logo.png",
fit: BoxFit.cover,
),
),
);
}
}
I hope it was a useful article. See you soon
Tag – Flutter Login Page UI, Login Page UI in Flutter, Login Signup Screen in Flutter
#iOS #Android #Flutter #dev