Hi developers hope you enjoy your development in flutter so we have create a new screen for UPI payment in flutter with card save and enter the UPI screen.
Custom App Bar
AppBar(
centerTitle: false,
leadingWidth: 30,
automaticallyImplyLeading: false,
title: Text(
'Payment',
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.whiteA703,
fontSize: getFontSize(
18,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w500,
),
),
backgroundColor: AppColors.headerColor,
leading: IconButton(
icon: const Icon(Icons.arrow_back, color: Colors.white),
onPressed: () => Navigator.of(context).pop(),
),
elevation: 0,
),
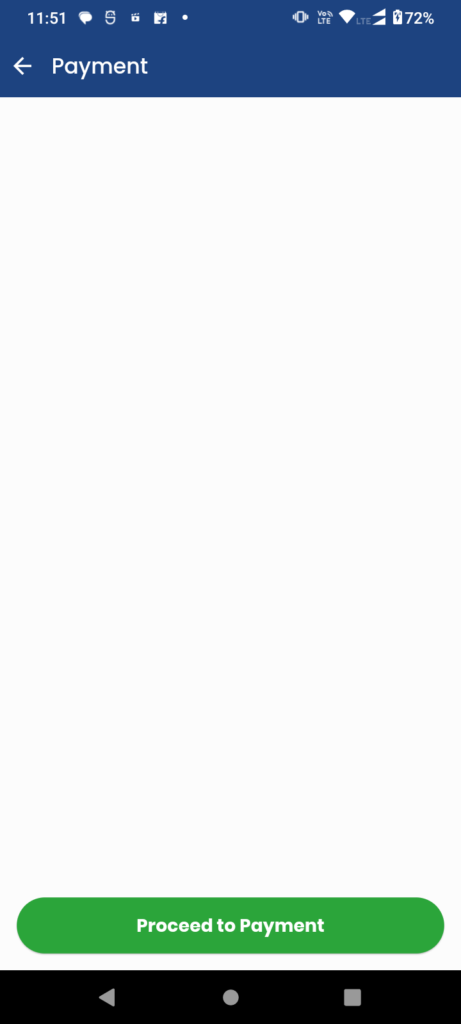
Amount Title
Row(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
const Padding(
padding: EdgeInsets.only(left: 13.0),
child: Text(
'Amount to Pay for order confirmation',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 14,
//fontWeight: FontWeight.bold,
color: AppColors.textPolicyBlackColor),
),
),
const Spacer(),
Container(
child: const Padding(
padding: EdgeInsets.only(left: 8.0, right: 32.0),
child: Text(
'₹ 9,300',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 16,
fontWeight: FontWeight.bold,
color: AppColors.textPolicyBlackColor),
),
),
),
//---2
],
),

Enter UPI Box
Container(
child: Column(
children: [
Container(
child: Row(
children: [
Expanded(
flex: 5,
child: Column(
crossAxisAlignment:
CrossAxisAlignment.start,
children: [
const Padding(
padding:
EdgeInsets.fromLTRB(13, 0, 0, 5),
child: Text(
"Enter UPI ID",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 14,
color: AppColors
.textPolicyBlackColor),
),
),
Padding(
padding:
const EdgeInsets.only(left: 13.0),
child: TextFormField(
style: TextStyle(
color: Colors.black,
fontSize: 14),
controller: upiController,
decoration: InputDecoration(
fillColor: ColorConstant.blue50,
filled: true,
alignLabelWithHint: true,
floatingLabelBehavior:
FloatingLabelBehavior.never,
contentPadding:
EdgeInsets.fromLTRB(
8, 5, 10, 5),
labelText: "",
border: OutlineInputBorder(
borderRadius:
BorderRadius.circular(2.0),
),
labelStyle: TextStyle(
color:
AppColors.textWaterColor,
fontSize: 12),
),
),
),
],
),
),
SizedBox(width: 8),
Expanded(
flex: 2,
child: Column(
crossAxisAlignment:
CrossAxisAlignment.start,
children: [
Padding(
padding: EdgeInsets.only(
left: 1, right: 1, top: 20),
child: Container(
padding: EdgeInsets.all(8.0),
// height: 100,
child: Expanded(
child: Container(
height: 50,
width: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor:
AppColors.blueBtnColor,
),
onPressed: () async {
//enter your code},
child: const Text(
"Verify",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle:
FontStyle.normal,
fontSize: 16,
color: Colors.white),
),
),
)),
),
),
],
),
),
],
),
),
],
),
),

Add Save UPI Card in your App
Container(
height: 165,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(1),
color: AppColors.policyCopenColor,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
listofUPI(upiArray),
],
),
),
Card ListView in flutter
Widget listofUPI(List<String> upiList) {
return Container(
width: double.infinity,
decoration: BoxDecoration(
color: ColorConstant.gray50,
boxShadow: [
BoxShadow(
color: ColorConstant.black90026,
spreadRadius: getHorizontalSize(
2.00,
),
blurRadius: getHorizontalSize(
2.00,
),
offset: const Offset(
0,
2,
),
),
],
),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.start,
children: [
Padding(
padding: getPadding(
left: 19,
top: 12,
right: 19,
),
child: Text(
"Your Saved UPI ID",
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.gray700,
fontSize: getFontSize(
12,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
Align(
alignment: Alignment.center,
child: Padding(
padding: getPadding(
left: 18,
top: 14,
right: 18,
bottom: 15,
),
child: ListView.builder(
padding: EdgeInsets.zero,
physics: BouncingScrollPhysics(),
controller: ScrollController(),
shrinkWrap: true,
itemCount: (upiList.length),
itemBuilder: (context, i) {
return InkWell(
enableFeedback: true,
onTap: () {
print(" Card index is $i");
},
child: Align(
alignment: Alignment.center,
child: Padding(
padding: getPadding(
top: 1.099991,
bottom: 2.099991,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: [
Padding(
padding: getPadding(
top: 9,
bottom: 9,
),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: [
SizedBox(
height: 16,
child: Radio(
value: i,
groupValue: valueChange,
onChanged: (value) {
setState(() {
valueChange = value as int;
print(value);
});
}),
),
Padding(
padding: getPadding(
left: 9,
top: 1,
bottom: 1,
),
child: Text(
upiList[i],
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.gray700,
fontSize: getFontSize(
14,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
Padding(
padding: getPadding(
left: 16,
top: 1,
bottom: 2,
),
child: Text(
"1947 xxxx xxxx 0906",
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.indigo800,
fontSize: getFontSize(
13,
),
fontFamily: 'Roboto',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
],
),
),
ClipRRect(
borderRadius: BorderRadius.circular(
getHorizontalSize(
1.09,
),
),
child: CommonImageView(
imagePath: ImageConstant.imgUPI,
height: getSize(
35.00,
),
width: getSize(
35.00,
),
fit: BoxFit.cover,
),
),
],
),
),
),
);
},
),
),
),
],
),
);
}
final List<String> upiArray = [
'Paytm',
'Gpay',
//--add more as per your need
];
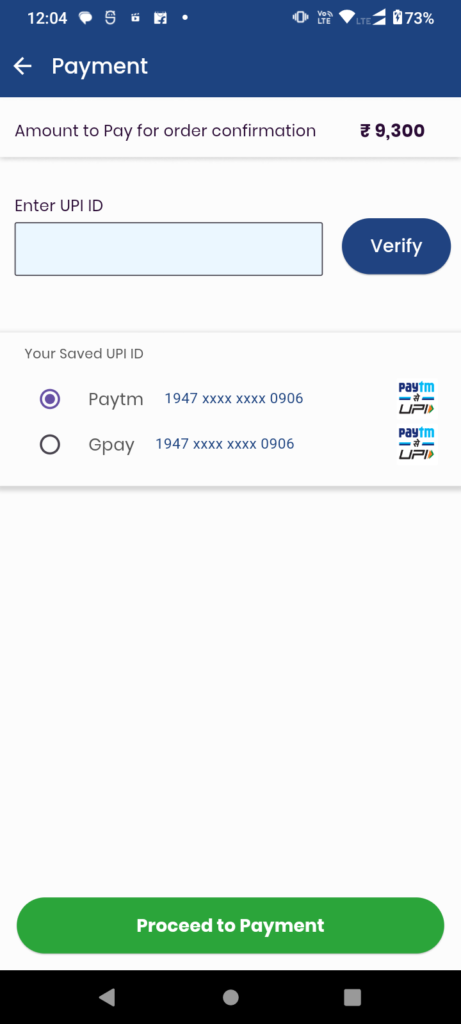
const SizedBox(
height: 108,
),
const Text(
"Rest assured your transaction is completely safe",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
//fontWeight: FontWeight.bold,
fontSize: 10,
color: AppColors.textPolicyBlackColor),
),
const SizedBox(
height: 5,
),
Container(
child: Image.asset(
'assets/images/g12.png',
fit: BoxFit.scaleDown,
),
),
const SizedBox(
height: 2,
),
const Text(
"32 bit secure transaction gateway",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 10,
color: AppColors.textPolicyBlackColor),
),
Output
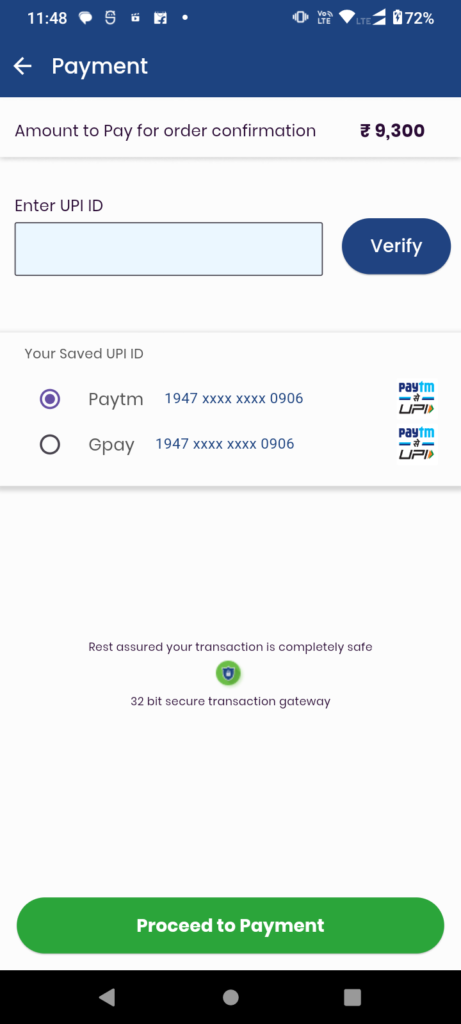
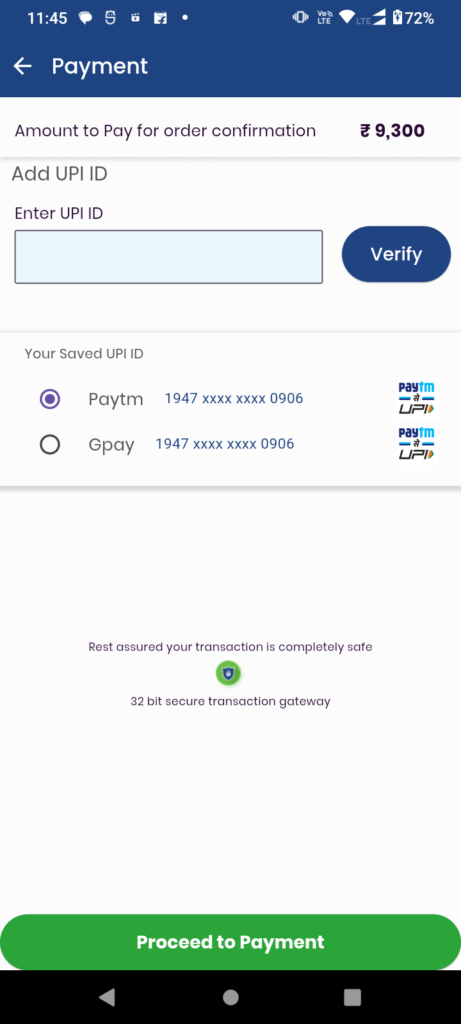
If any question and query please mail – mukesh.wrms@gmail.com