Colors in flutter
Colors are used to style and customize(customize app theme) various user interface elements such as widgets, text, and backgrounds. The Colors class in Flutter provides a variety of predefined colors that can be used directly in code, such as Colors.red, Colors.blue, Colors.green, and so on.

In addition to the predefined colors, you can also define custom colors using a combination of red, green, and blue values or by specifying a hexadecimal value. These custom colors can then be used in your app to give it a unique look and feel.
Example:- Login button color code and output image (iPhone 14 plus)
static Color green300 = fromHex('#36b3a8');
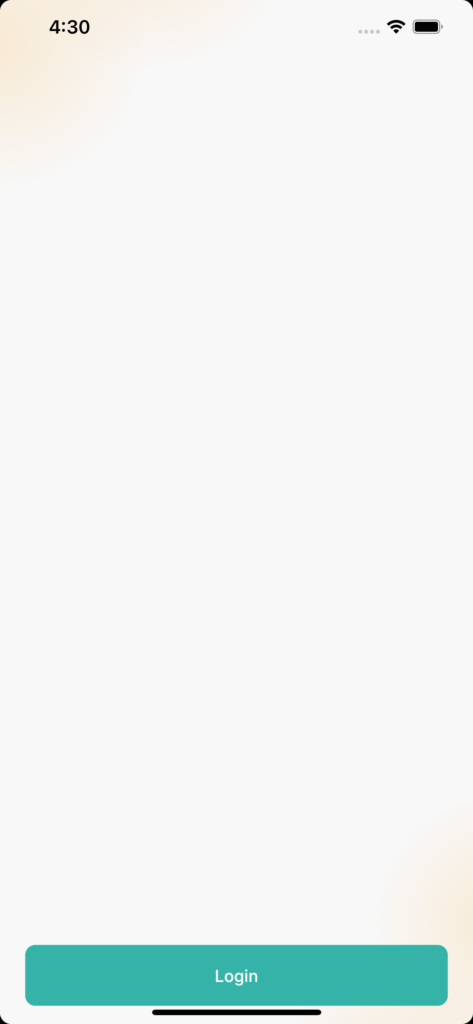
Hexadecimal code
Colors are represented using a Color object. One way to create a Color object is by specifying its red, green, blue, and alpha components using values between 0 and 255.
Example: – Color(0, 21, 255) represents the color blue with full opacity.
import 'package:flutter/material.dart';
Color getDynamicColor(String colorsCode) {
Color finalColorCode;
if (colorsCode.length == 6) {
var col = '0xFF' + colorsCode;
var intCol = int.parse(col);
Color finalCol = Color(intCol);
finalColorCode = finalCol;
} else {
var col = colorsCode;
var intCol = int.parse(col);
Color finalCol = Color(intCol);
finalColorCode = finalCol;
}
return finalColorCode;
}
Splash screen(login)
import 'package:flutter/material.dart';
class SplashScreen extends StatelessWidget {
final AssetImage backgroundImageLocal = AssetImage('assets/images/bg.png');
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black,
body: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: backgroundImageLocal,
fit: BoxFit.fill,
),
borderRadius: BorderRadius.circular(10),
),
width: double.maxFinite,
padding: getPadding(left: 17, top: 15, right: 17, bottom: 15),
child: Column(mainAxisAlignment: MainAxisAlignment.end, children: [
Spacer(),
CustomButton(
height: getVerticalSize(50),
text: "Login",
margin: getMargin(left: 3, top: 87, right: 3),
variant: ButtonVariant.FillBluegray50,
onTap: () => onTapLogin(context))
])),
);
}
onTapLogin(BuildContext context) {
// TODO: implement Actions
//.Your implementation
}
}
Another way to create a Color object is by specifying its hexadecimal value. Hexadecimal colors are represented by a hash symbol followed by six hexadecimal digits, where the first two digits represent the red component, the next two represent the green component, and the last two represent the blue component. For example, the color red can be represented as a Color(0xFFFF0000) in hexadecimal.
To use a hexadecimal color in Flutter, simply create a Color object with the desired hexadecimal value. For example, to use the color red in hexadecimal, you can write Color(0xFFFF0000).