To draw a rectangle on a Google Map in a Flutter app, you’ll need to use the google_maps_flutter package, which allows you to integrate Google Maps into your Flutter application. Here are the steps to create a Flutter app with a Google Map that displays a rectangle:
Create a new Flutter project or open an existing one.
Add the google_maps_flutter package to your pubspec.yaml file:
dependencies:
flutter:
sdk: flutter
google_maps_flutter: ^2.5.0
Run this command:
With Flutter:
flutter pub add google_maps_flutter
Import it
Now in your Dart code, you can use:
import 'package:google_maps_flutter/google_maps_flutter.dart';
import 'package:flutter/material.dart';
Running Steps
- Run flutter pub get to fetch the package.
- Ensure you have the required permissions for location access. You’ll need to add the following permissions to your AndroidManifest.xml file (located in android/app/src/main):
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
Create a stateful widget for your Google Map Screen.
class ShowMap extends StatefulWidget {
final List<String>? pps;
final List<String>? ppl;
final double? getCurrentLet;
final double? getCurrentLong;
final List<LatLng>? latlngList;
ShowMap({
Key? key,
this.pps,
this.ppl,
this.getCurrentLet,
this.getCurrentLong,
this.latlngList,
}) : super(key: key);
@override
_ShowMapState createState() => _ShowMapState();
}
class _ShowMapState extends State<ShowMap> {
LatLng? centerLetLong;
Position? currentLocation;
double? setCurrentLet;
double? setCurrentLong;
final Set<Polyline> _polylines = {};
bool isShowPass = false;
bool onError = false;
final nameController = TextEditingController();
//---------end map key----------
Completer<GoogleMapController> mapController = Completer();
CameraPosition? cameraPosition;
String location = "Enter your location";
Set<Polygon> _polygon = HashSet<Polygon>();
Set<Marker> _markers = Set<Marker>();
double? mapCenterLat = 26.4499;
double? mapCenterLong = 80.3319;
List<LatLng> points = [];
final Set<Polyline> polyline = {};
var markerIds = HashMap<String, MarkerId>();
final markers = <MarkerId, Marker>{};
@override
void initState() {
super.initState();
ploy();
getUserLocation();
}
Future<Position> locateUser() async {
return Geolocator.getCurrentPosition(
desiredAccuracy: LocationAccuracy.high);
}
LocationPermission? permission;
getUserLocation() async {
permission = await Geolocator.requestPermission();
currentLocation = await locateUser();
setState(() {
centerLetLong =
LatLng(currentLocation!.latitude, currentLocation!.longitude);
setCurrentLet = currentLocation!.latitude;
setCurrentLong = currentLocation!.longitude;
});
}
List<LatLng> pointData = [];
LatLngBounds computePoliyLine(List<LatLng> list) {
pointData.clear();
double s, n, w, e;
assert(list.isNotEmpty);
for (var i = 0; i < list.length; i++) {
var latlng1 = list[i];
var s = latlng1.latitude,
n = latlng1.latitude,
w = latlng1.longitude,
e = latlng1.longitude;
double yMin, yMax, xMin, xMax;
for (var i = 1; i < list.length; i++) {
var latlng = list[i];
s = min(s, latlng.latitude);
n = max(n, latlng.latitude);
w = min(w, latlng.longitude);
e = max(e, latlng.longitude);
var ymin_xmin = LatLng(s, e);
var ymin_xmax = LatLng(s, w);
var ymax_xmax = LatLng(n, w);
var ymax_xmin = LatLng(n, e);
pointData.add(ymin_xmin);
pointData.add(ymin_xmax);
pointData.add(ymax_xmax);
pointData.add(ymax_xmin);
pointData.add(ymin_xmin);
}
}
return LatLngBounds(
southwest: LatLng(25.72, 91.19), northeast: LatLng(25.72, 91.19));
}
List<LatLng> points = [
LatLng(25.97, 90.94),
LatLng(25.72, 91.19),
LatLng(25.97, 91.19),
LatLng(25.72, 91.44),
LatLng(25.97, 91.44),
LatLng(25.72, 91.69),
LatLng(25.97, 91.69),
LatLng(25.72, 91.94),
LatLng(26.22, 90.94),
LatLng(25.97, 91.19),
LatLng(26.22, 91.19),
LatLng(25.97, 91.44),
LatLng(26.22, 91.44),
LatLng(25.97, 91.69),
LatLng(26.22, 91.69),
LatLng(25.97, 91.94),
LatLng(26.47, 90.94),
LatLng(26.22, 91.19),
LatLng(26.47, 91.19),
LatLng(26.22, 91.44),
LatLng(26.47, 91.44),
LatLng(26.22, 91.69),
LatLng(26.47, 91.69),
LatLng(26.22, 91.94),
];
Future<void> ploy() async {
computeBounds(widget.latlngList!);
final Uint8List redMarker =
await getBytesFromAsset('assets/images/location_markar.png', 33);
var redIcon = BitmapDescriptor.fromBytes(redMarker);
for (LatLng loc in points!) {
polyline.add(Polyline(
polylineId: PolylineId('name'.toString()),
visible: true,
points: pointData,
color: Colors.blue,
width: 3,
));
}
}
Future<Uint8List> getBytesFromAsset(String path, int width) async {
ByteData data = await rootBundle.load(path);
ui.Codec codec = await ui.instantiateImageCodec(data.buffer.asUint8List(),
targetWidth: width);
ui.FrameInfo fi = await codec.getNextFrame();
return (await fi.image.toByteData(format: ui.ImageByteFormat.png))!
.buffer
.asUint8List();
}
@override
Widget build(BuildContext context) {
return Scaffold(
//drawer: myDrawer(),
appBar: AppBar(
centerTitle: false,
leadingWidth: 50,
automaticallyImplyLeading: false,
title: Text(
'Source Info',
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.center,
style: TextStyle(
color: ColorConstant.whiteA703,
fontSize: getFontSize(
16,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w500,
),
),
leading: IconButton(
icon: const Icon(Icons.arrow_back, color: Colors.white),
onPressed: () => Navigator.of(context).pop(),
),
backgroundColor: AppColors.headerColor,
),
backgroundColor: AppColors.mainBg,
body: Container(
child: Stack(children: [
GoogleMap(
myLocationEnabled: true,
myLocationButtonEnabled: true,
zoomControlsEnabled: true,
scrollGesturesEnabled: true,
markers: _markers,
polylines: polyline,
initialCameraPosition: CameraPosition(
target: LatLng(widget.getCurrentLet!, widget.getCurrentLong!),
zoom: 7.6, //initial zoom level
),
mapType: MapType.normal, //map type
onMapCreated: (GoogleMapController controller) {
mapController.complete(controller);
},
),
Center(
//picker image on google map
child: InkWell(
child: Padding(
padding: const EdgeInsets.only(left: 5.0),
child: SizedBox(
height: 40,
child: SvgPicture.asset("assets/images/location_ic.svg",
fit: BoxFit.scaleDown),
),
),
onTap: () {}),
),
);
}
}
Output


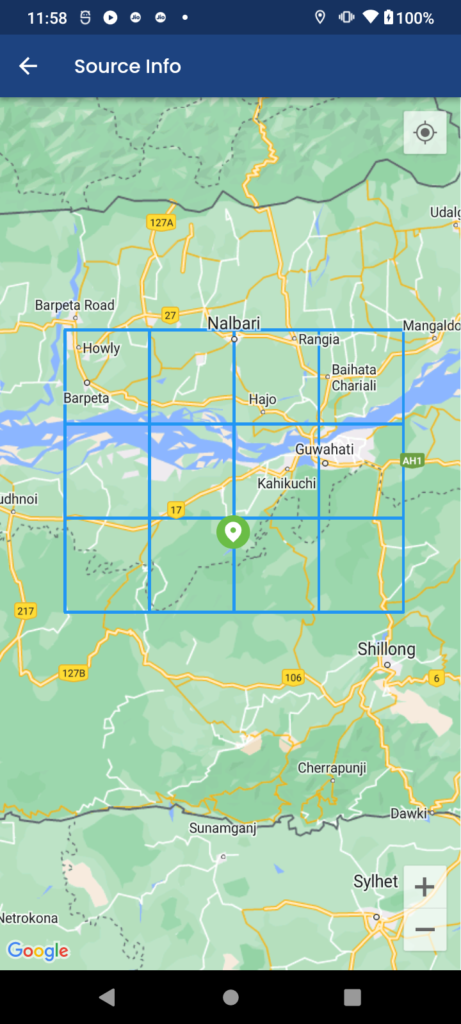
- GoogleMap is the widget responsible for displaying the map.
- initialCameraPosition defines the initial center and zoom level of the map.
- Polylines is used to draw the rectangle. You define the polylines points, stroke width, stroke color, and fill color to customize its appearance.
Make sure to replace the coordinates in LatLng with your desired rectangle’s bounds.