
Flutter UPI Payment Screen
Hello developers, today I have selected a very important topic in flutter app development, day to day we use the payment option in over mobile app so it’s a very important aspect to create a screen in a flutter developer very easy way and beautiful layout of the screen.
This topic covers –
- how to create a UPI Payment Integration Screen?
- how do you implement UPI payment in flutter?
- payment gateway integration with flutter?
- adding UPI payment screen in flutter?
- flutter listview.builder displaying list item?
- binding listview in flutter?
- flutter display list items with array data?
- how do you bind data in Flutter?
- how do you use itemCount in Flutter?
- how do I use ListView separated in Flutter?
- how can you update a ListView dynamically Flutter?
- Radio button add in list view and select only one item?
Running app Video on android device
Let’s get started on the main steps!
Please see the if you don’t know the flutter basics things please flow our previous articles have already declared the use environment, project structure, and pod (pub file links).
UPI Page Widget initilaztion
int selectedIndex = 0;
int valueChange = 0;
onSelected(int index) {
setState(() => selectedIndex = index);
}
final List<String> upiArray = [
'Paytm',
'Gpay',
];
UPI Screen Top level
Container(
height: 60,
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("your image link"),
fit: BoxFit.fill,
),
),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
const Padding(
padding: EdgeInsets.only(left: 13.0),
child: Text(
'Amount to Pay for order confirmation',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 14,
color: AppColors.textPolicyBlackColor),
),
),
const Spacer(),
Container(
child: const Padding(
padding: EdgeInsets.only(left: 8.0, right: 32.0),
child: Text(
'₹ 9,300',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 16,
fontWeight: FontWeight.bold,
color: AppColors.textPolicyBlackColor),
),
),
),
],
),
),
AppColors:
Please used App Colors Code on our previous articles.
UPI Layout

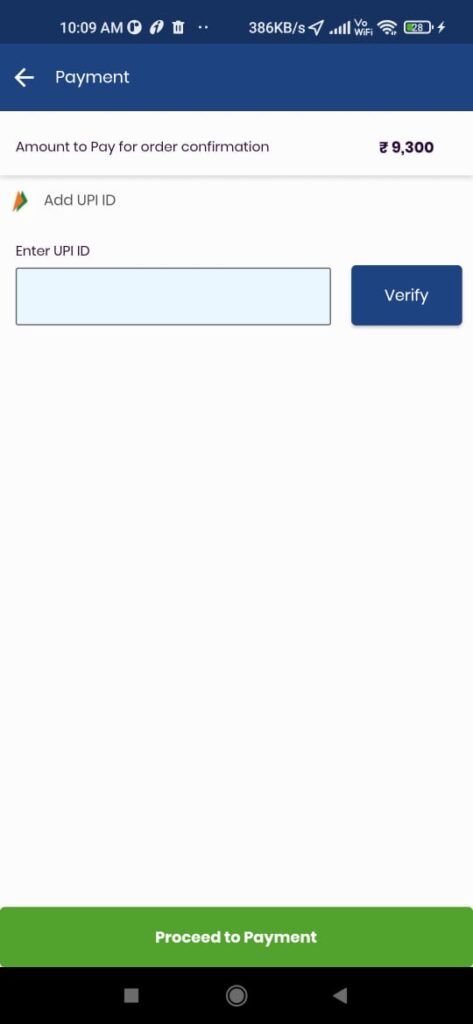
2nd Container UPI Screen
Container(
height: 150,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(1),
color: AppColors.policyCopenColor,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Row(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
InkWell(
child: Padding(
padding: const EdgeInsets.all(5.0),
child: SizedBox(
height: 20,
child:
Image.asset('your images ',
fit: BoxFit.scaleDown),
),
),
onTap: () {}),
Padding(
padding: const EdgeInsets.only(left: 2.0),
child: Text(
'Add UPI ID ',
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.gray700,
fontSize: getFontSize(
16,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
//---2
],
),
],
),
SizedBox(
height: 10,
),
Container(
child: Column(
children: [
Container(
child: Row(
children: [
Expanded(
flex: 5,
child: Column(
crossAxisAlignment:
CrossAxisAlignment.start,
children: [
const Padding(
padding:
EdgeInsets.fromLTRB(13, 0, 0, 5),
child: Text(
"Enter UPI ID",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 14,
color: AppColors
.textPolicyBlackColor),
),
),
Padding(
padding:
const EdgeInsets.only(left: 13.0),
child: TextFormField(
style: TextStyle(
color: Colors.black,
fontSize: 14),
controller: upiController,
decoration: InputDecoration(
fillColor: ColorConstant.blue50,
filled: true,
alignLabelWithHint: true,
floatingLabelBehavior:
FloatingLabelBehavior.never,
contentPadding:
EdgeInsets.fromLTRB(
8, 5, 10, 5),
labelText: "",
border: OutlineInputBorder(
borderRadius:
BorderRadius.circular(2.0),
),
labelStyle: TextStyle(
color:
AppColors.textWaterColor,
fontSize: 12),
),
),
),
],
),
),
SizedBox(width: 8),
Expanded(
flex: 2,
child: Column(
crossAxisAlignment:
CrossAxisAlignment.start,
children: [
Padding(
padding: EdgeInsets.only(
left: 1, right: 1, top: 20),
child: Container(
padding: EdgeInsets.all(8.0),
child: Expanded(
child: Container(
height: 50,
width: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary:
AppColors.blueBtnColor,
onPrimary: Colors.white,
side: const BorderSide(
color: AppColors
.blueBtnColor,
width: 10),
),
onPressed: () async {},
child: const Text(
"Verify",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle:
FontStyle.normal,
fontSize: 16,
color: Colors.white),
),
),
)),
),
),
],
),
),
],
),
),
],
),
),
const SizedBox(
height: 15,
),
],
),
),
3rd Container UPI Screen
Container(
height: 165,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(1),
color: AppColors.policyCopenColor,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
listofUPI(upiArray),
],
),
),
Save UPI List Widget(ListView Bind with radio button)
Widget listofUPI(List<String> upiList) {
return Container(
width: double.infinity,
decoration: BoxDecoration(
color: ColorConstant.gray50,
boxShadow: [
BoxShadow(
color: ColorConstant.black90026,
spreadRadius: getHorizontalSize(
2.00,
),
blurRadius: getHorizontalSize(
2.00,
),
offset: const Offset(
0,
2,
),
),
],
),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.start,
children: [
Padding(
padding: getPadding(
left: 19,
top: 12,
right: 19,
),
child: Text(
"Your Saved UPI ID",
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.gray700,
fontSize: getFontSize(
12,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
Align(
alignment: Alignment.center,
child: Padding(
padding: getPadding(
left: 18,
top: 14,
right: 18,
bottom: 15,
),
child: ListView.builder(
padding: EdgeInsets.zero,
physics: BouncingScrollPhysics(),
controller: ScrollController(),
shrinkWrap: true,
itemCount: (upiList.length),
itemBuilder: (context, i) {
return InkWell(
enableFeedback: true,
onTap: () {
print(" Card index is $i");
},
child: Align(
alignment: Alignment.center,
child: Padding(
padding: getPadding(
top: 1.099991,
bottom: 2.099991,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: [
Padding(
padding: getPadding(
top: 9,
bottom: 9,
),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: [
SizedBox(
height: 16,
child: Radio(
value: i,
groupValue: valueChange,
onChanged: (value) {
setState(() {
valueChange = value as int;
print(value);
});
}),
),
Padding(
padding: getPadding(
left: 9,
top: 1,
bottom: 1,
),
child: Text(
upiList[i],
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.gray700,
fontSize: getFontSize(
14,
),
fontFamily: 'Poppins',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
Padding(
padding: getPadding(
left: 16,
top: 1,
bottom: 2,
),
child: Text(
"1947 xxxx xxxx 0906",
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.left,
style: TextStyle(
color: ColorConstant.indigo800,
fontSize: getFontSize(
13,
),
fontFamily: 'Roboto',
fontWeight: FontWeight.w400,
height: 1.00,
),
),
),
],
),
),
ClipRRect(
borderRadius: BorderRadius.circular(12),
child:
ImageView(
imagePath: ImageInfo.imgUPI,
height: getSize(
35.00,
),
width: getSize(
35.00,
),
fit: BoxFit.cover,
),
),
],
),
),
),
);
},
),
),
),
],
),
);
}
Note: getSize, getFontSize, getPadding, getHorizontalSize as per your need to change it.


Other Container UPI Screen
const SizedBox(
height: 108,
),
const Text(
"Rest assured your transaction is completely safe",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 10,
color: AppColors.textPolicyBlackColor),
),
const SizedBox(
height: 5,
),
Container(
child: Image.asset(
'your images',
fit: BoxFit.scaleDown,
),
),
const SizedBox(
height: 2,
),
//----add lable bottom of UPI container
const Text(
"32 bit secure transaction gateway",
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontSize: 10,
color: AppColors.textPolicyBlackColor),
),
final output

Conclusion:
In this article, we discussed the most valuable part of the UPI Payment Screen text form and separated the container, and bind the list view with a radio button, to make our development life easier In future parts, I will share some important code of UPI payment screen to make your Flutter Development journey a bit faster and many tips related to Flutter and Dart.
I hope it was a useful article, please share and subscribe to my channel, You have enjoyed the most. Thanks for reading and if you have any questions or comments, See you soon.