To get the current location and address using Google Maps in Flutter, you can used the geolocator and geocoding packages.
Have following some step by step process google map create.
I)-Add the necessary dependencies to your pubspec.yaml file: Here’s an example of how you can achieve this:
dependencies:
geolocator: ^9.0.2
geocoding: ^2.1.0
- Run flutter pub get to fetch the packages.
- Import the required packages in your Dart file:
import 'package:geolocator/geolocator.dart';
import 'package:geocoding/geocoding.dart';
Define a method to fetch the current location and address:
LocationPermission? permission;
LatLng? centerLetLong;
Position? currentLocation;
double? setCurrentLet;
double? setCurrentLong;
String googleApikey = 'your google map key';
@override
void initState() {
super.initState();
getUserLocation();
}
Future<Position> locateUser() async {
return Geolocator.getCurrentPosition(
desiredAccuracy: LocationAccuracy.high);
}
getUserLocation() async {
permission = await Geolocator.requestPermission();
if (widget.userType == 'gustUser') {
currentLocation = await locateUser();
print(currentLocation);
setState(() {
centerLetLong =
LatLng(currentLocation!.latitude, currentLocation!.longitude);
setCurrentLet = currentLocation!.latitude;
setCurrentLong = currentLocation!.longitude;
});
print('userCurentLocation $centerLetLong');
} else {
currentLocation = await locateUser();
setState(() {
centerLetLong =
LatLng(currentLocation!.latitude, currentLocation!.longitude);
setCurrentLet = currentLocation!.latitude;
setCurrentLong = currentLocation!.longitude;
});
print('userCurentLocation $centerLetLong');
}
}
Google Map Example more info.
Container(
child: Stack(children: [
GoogleMap(
myLocationEnabled: true,
myLocationButtonEnabled: true,
zoomControlsEnabled: true,
scrollGesturesEnabled: true,
initialCameraPosition: CameraPosition(
//target: LatLng(setCurrentLet!, setCurrentLong!),
target: LatLng(widget.getCurrentLet!.toDouble(),
widget.getCurrentLong!.toDouble()), //initial position
zoom: 14.0, //initial zoom level
),
mapType: MapType.normal,
onMapCreated: (controller) {
setState(() {
mapController = controller;
});
},
onCameraMove: (CameraPosition cameraPositiona) {
cameraPosition = cameraPositiona;
},
onCameraIdle: () async {
List<Placemark> placemarks = await placemarkFromCoordinates(
cameraPosition!.target.latitude,
cameraPosition!.target.longitude);
setState(() {
location = placemarks.first.name.toString() +
", " +
placemarks.first.subLocality.toString() +
',' +
placemarks.first.locality.toString() +
',' +
placemarks.first.administrativeArea.toString() +
',' +
placemarks.first.postalCode.toString() +
',' +
placemarks.first.country.toString();
});
},
),
Center(
//picker image on google map
child: InkWell(
child: Padding(
padding: const EdgeInsets.only(left: 5.0),
child: SizedBox(
height: 40,
child: SvgPicture.asset("assets/images/location_ic.svg",
fit: BoxFit.scaleDown),
),
),
onTap: () {}),
),
//search autoconplete input
Positioned(
//search input bar
child: InkWell(
onTap: () async {
var place = await PlacesAutocomplete.show(
context: context,
apiKey: googleApikey,
mode: Mode.overlay,
types: [],
strictbounds: false,
components: [Component(Component.country, 'in')],
//google_map_webservice package
onError: (err) {
print(err);
});
if (place != null) {
setState(() {
location = place.description.toString();
});
//form google_maps_webservice package
final plist = GoogleMapsPlaces(
apiKey: googleApikey,
apiHeaders: await GoogleApiHeaders().getHeaders(),
//from google_api_headers package
);
String placeid = place.placeId ?? "0";
final detail = await plist.getDetailsByPlaceId(placeid);
final geometry = detail.result.geometry!;
final lat = geometry.location.lat;
final lang = geometry.location.lng;
var newlatlang = LatLng(lat, lang);
//move map camera to selected place with animation
mapController?.animateCamera(
CameraUpdate.newCameraPosition(
CameraPosition(target: newlatlang, zoom: 15)));
}
},
child: Container(
color: AppColors.searchLocationMapColor,
child: Card(
child: Container(
color: AppColors.searchLocationMapColor,
width: MediaQuery.of(context).size.width,
child: ListTile(
leading: InkWell(
child: Padding(
padding: const EdgeInsets.only(left: 5.0),
child: SizedBox(
height: 40,
child: SvgPicture.asset(
"assets/images/location_ic.svg",
fit: BoxFit.scaleDown),
),
),
onTap: () async {
var place = await PlacesAutocomplete.show(
context: context,
apiKey: googleApikey,
mode: Mode.overlay,
types: [],
strictbounds: false,
components: [
Component(Component.country, 'in')
],
//google_map_webservice package
onError: (err) {
print(err);
});
if (place != null) {
setState(() {
location = place.description.toString();
});
//form google_maps_webservice package
final plist = GoogleMapsPlaces(
apiKey: googleApikey,
apiHeaders:
await GoogleApiHeaders().getHeaders(),
//from google_api_headers package
);
String placeid = place.placeId ?? "0";
final detail = await plist
.getDetailsByPlaceId(placeid);
final geometry = detail.result.geometry!;
final lat = geometry.location.lat;
final lang = geometry.location.lng;
var newlatlang = LatLng(lat, lang);
//move map camera to selected place with animation
mapController?.animateCamera(
CameraUpdate.newCameraPosition(
CameraPosition(
target: newlatlang, zoom: 15)));
}
}),
title: Text(
location,
style: const TextStyle(
color: Colors.black, fontSize: 16),
),
dense: true,
)),
),
)))
]),
),
Output
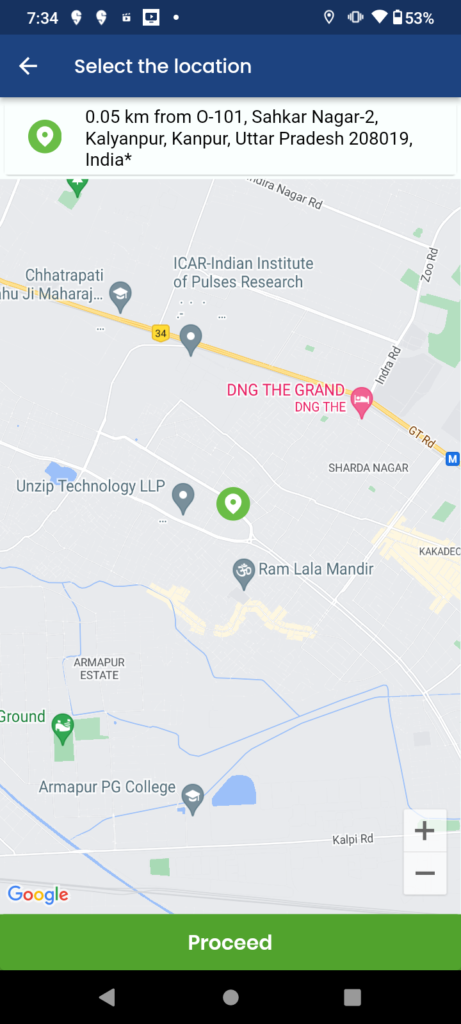
Thank you.
May I request that you elaborate on that? Your posts have been extremely helpful to me. Thank you!
You helped me a lot by posting this article and I love what I’m learning.
Thank you