Are you struggling to align text in your Flutter application? Do you want to make your UI more appealing and professional? In this article, we will discuss how to set text-align Flutter and explore the different options available to you. We will cover everything from the basics to advanced techniques, so whether you are a beginner or an experienced developer, you will find this guide helpful.
How to Set Text Align Flutter?
Aligning text in Flutter is quite simple and can be done using various properties. Here is how you can set text-align Flutter.
Using the TextAlign Property
The TextAlign property is used to align text horizontally within a Text widget. It can be set to one of the following values:
- left: Aligns text to the left.
- center: Centers text horizontally.
- right: Aligns text to the right.
- justify: Distributes text evenly between the left and right edges.
Example of how to use the TextAlign property:
Left Text Code
Align(
alignment: Alignment.centerLeft,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Center',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
Right Text Code
Align(
alignment: Alignment.centerRight,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Center',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
Center Screen Text
Container(
padding: EdgeInsets.zero,
decoration: BoxDecoration(border: Border.all()),
child: Align(
alignment: Alignment.center,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Center',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
),
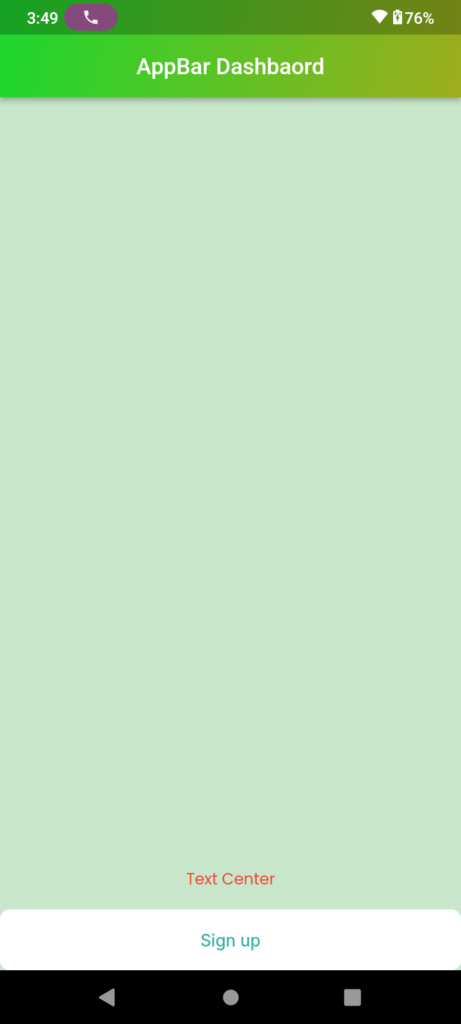

Center Right Text
Container(
padding: EdgeInsets.zero,
decoration: BoxDecoration(border: Border.all()),
child: Align(
alignment: Alignment.centerRight,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Right',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
),
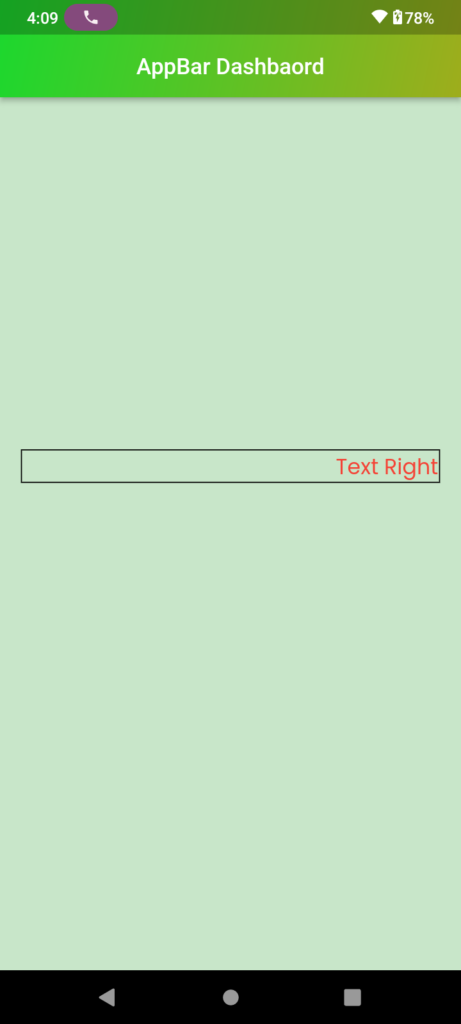
Justify Text
Container(
padding: EdgeInsets.zero,
decoration: BoxDecoration(border: Border.all()),
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Justify',
textAlign: TextAlign.justify,
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
)
),
),
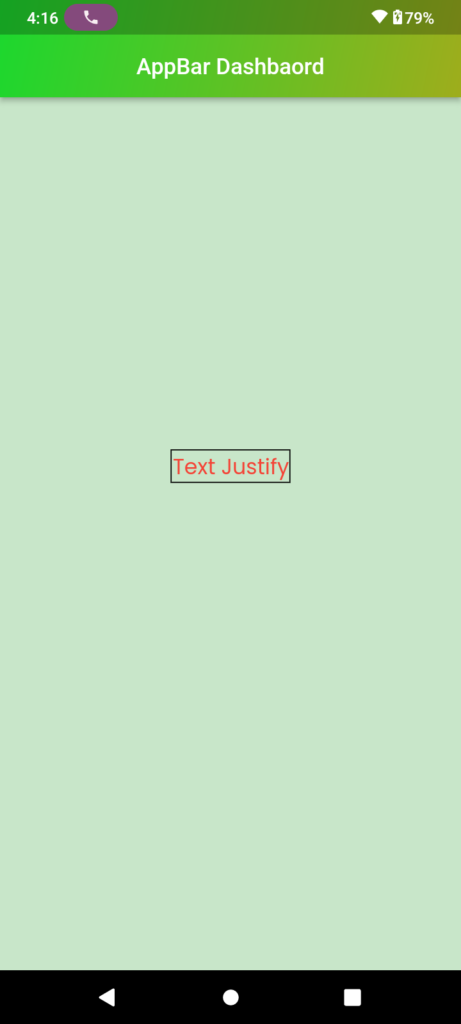
Using the VerticalAlign Property
The VerticalAlign property is used to align text vertically within a Text widget.
- top: Align text to the top.
- center: Centers text vertically.
- bottom: Aligns text to the bottom.
how to use the VerticalAlign property:
Other Text Alignment Options
Apart from the above-mentioned properties, there are other ways to align text in Flutter. Let’s check :
Fullter Floating Action Button
how to create a Floating Action Button in a flutter
Using the CrossAxisAlignment Property
The CrossAxisAlignment property is used to align widgets horizontally within a Row or a Column. Use values:
- start: Align widgets to the start edge of the container.
- end: Aligns widgets to the end edge of the container.
- center: Centers widgets horizontally within the container.
how to use the CrossAxisAlignment property:
Start Position in CrossAxisAlignment
"Main Line - mainAxisAlignment: MainAxisAlignment.start"
Container(
width: double.maxFinite,
padding: getPadding(top: 15),
child:
Column(mainAxisAlignment: MainAxisAlignment.start, children: [
// Spacer(),
Container(
padding: EdgeInsets.zero,
decoration: BoxDecoration(border: Border.all()),
child: Align(
alignment: Alignment.center,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Alignment in Top',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
),
])),
End Position in CrossAxisAlignment
"Main Line - mainAxisAlignment: MainAxisAlignment.end"
Container(
width: double.maxFinite,
padding: getPadding(top: 15),
child:
Column(mainAxisAlignment: MainAxisAlignment.end, children: [
// Spacer(),
Container(
padding: EdgeInsets.zero,
decoration: BoxDecoration(border: Border.all()),
child: Align(
alignment: Alignment.center,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Alignment in End',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
),
])),
Center Position in CrossAxisAlignment
Container(
width: double.maxFinite,
padding: getPadding(top: 15),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
// Spacer(),
Container(
padding: EdgeInsets.zero,
decoration: BoxDecoration(border: Border.all()),
child: Align(
alignment: Alignment.center,
child: Padding(
padding: EdgeInsets.only(left: 1.0),
child: Text(
'Text Alignment in Center',
style: TextStyle(
fontFamily: 'Poppins',
fontStyle: FontStyle.normal,
fontWeight: FontWeight.w500,
fontSize: 19,
color: Colors.red),
),
),
),
),
])),
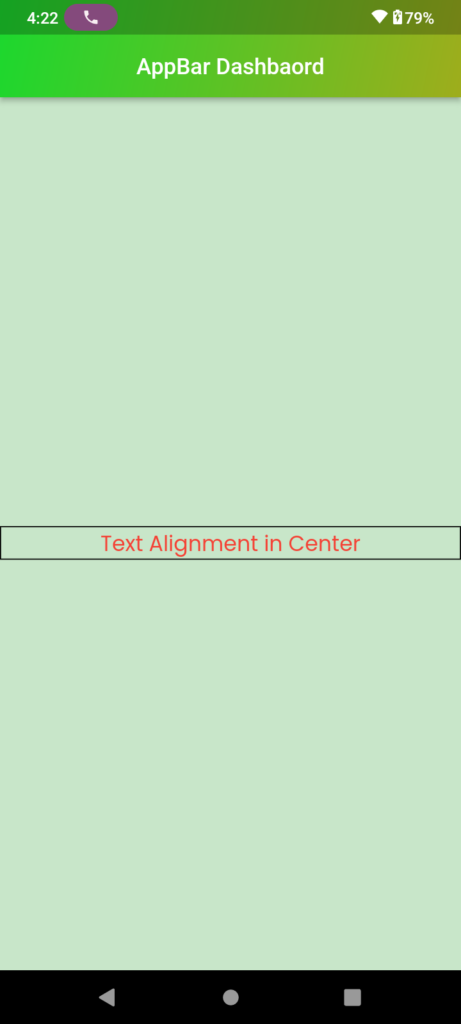

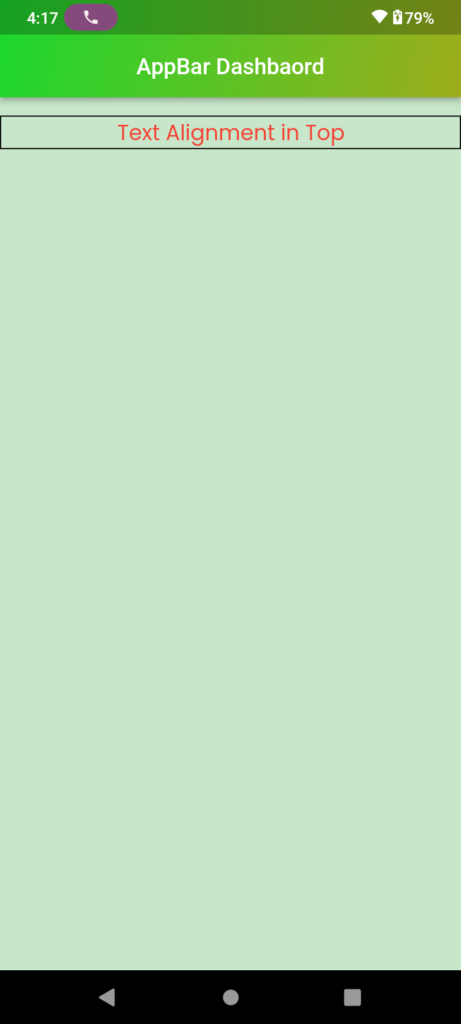
Frequently Asked Questions (FAQs)
Q1. How can I align text to the right in Flutter?
To align text to the right in Flutter, you can set the TextAlign property to TextAlign.right.
Q2. How can I align text to the center in Flutter?
To align text to the center in Flutter, you can set the TextAlign property to TextAlign.center.