This project is practicing making an IOS app Screen on how to create a List view in iOS | TableView.
In iOS app development every app needs the list of the show user data per you needed, I have to create a list view in the swift language used and implement the UI with API data. And binding list view with responses on a screen. if you learn the login screen in the flutter app.

What is a TableView in swift?
Table views in iOS display rows of vertically scrolling content in a single column. Each row in the table contains one piece of your app’s content. For example, the Contacts app displays the name of each contact in a separate row, and the main page of the Settings app shows the available groups of settings. You can configure a table to display a single long list of rows, or you can group related rows into sections to make navigating the content more accessible. Tables are common in apps with highly structured or organized hierarchically data. more info.
Swift Programming:- Swift is a general-purpose, multi-paradigm, compiled programming language developed by Apple Inc. and the open-source community.
Environment
- MacBook Air / Pro
- XCODE
- Swift programming
- iOS simulator 15+ Version
- Build iOS
- Run iPhone 11 Pro
- Terminal (on Mac)
Features structure
- MVVM structure
- Data module
- TableView UI Design
- Delegate pass value
Pods
- SwiftyJSON
- Alamofire
- GoogleMaps
- Charts
- JJFloatingActionButton
Let’s get started on the main steps!
Need some pods imported on the ViewController.
import UIKit
import SwiftyJSON
import Alamofire
import GoogleMaps
UIKit – The UIKit framework provides the core objects that you need to build apps for iOS and tvOS. You use these objects to display your content onscreen, interact with that content, and manage interactions with the system for References.
SwiftyJSON
SwiftyJSON is a library that helps to read and process JSON data from an API/Server.
Alamofire
Alamofire is an HTTP networking library written in Swift. It provides an elegant interface on top of Apple’s Foundation networking stack that simplifies common networking tasks for iOS and macOS for more info.
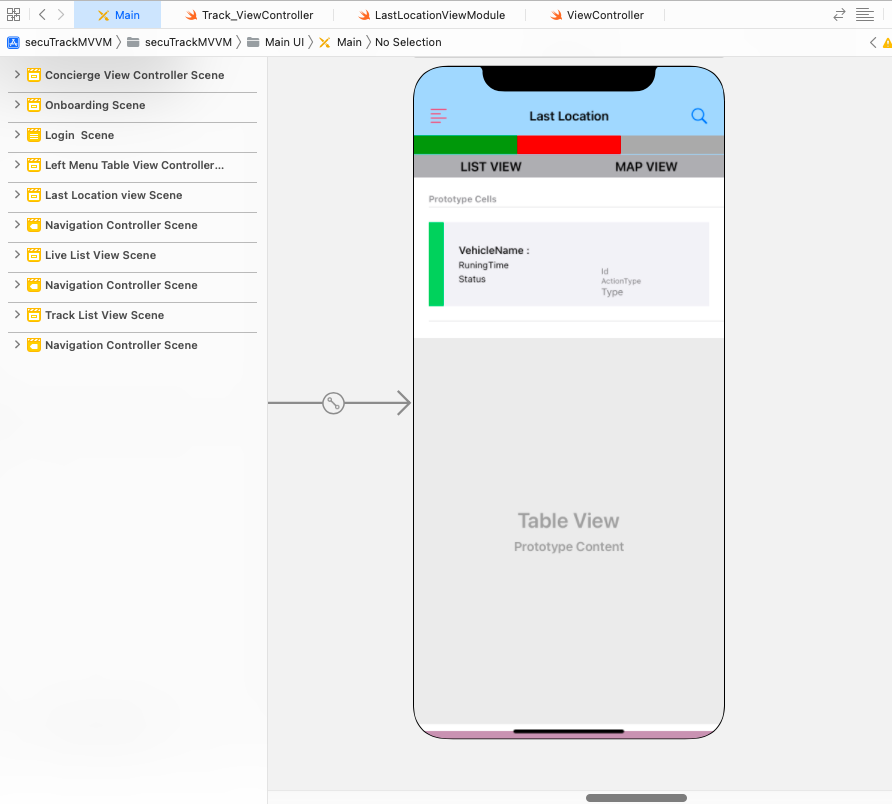
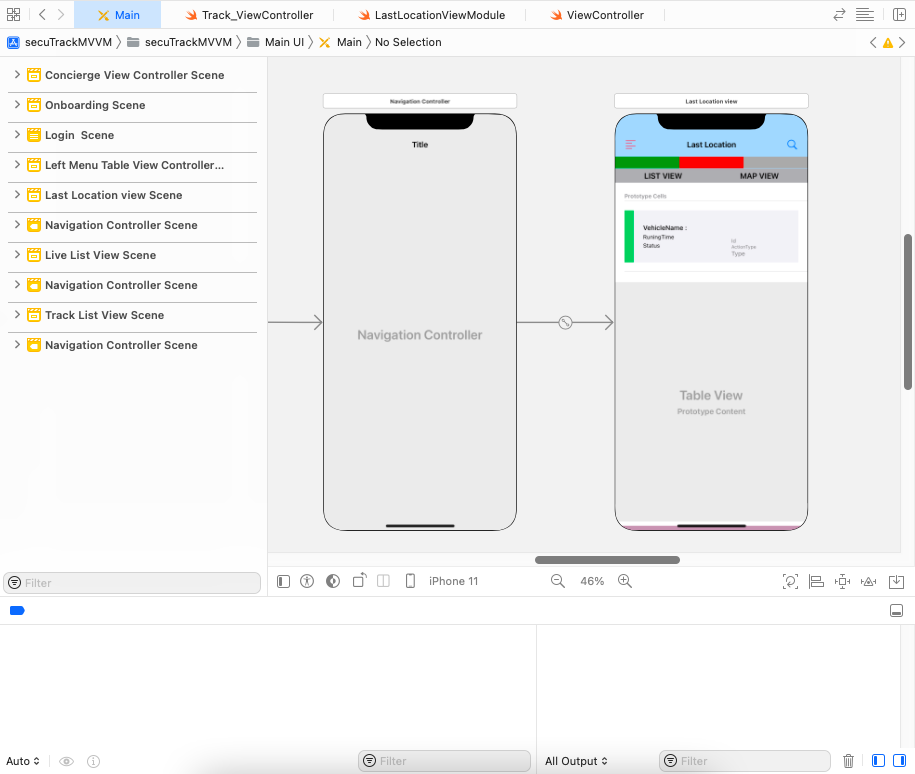
Main Code Vehicle model class-model folder
import Foundation
class VehicleArray: Codable {
let status,
let vehicleList: [VehicleList]?
enum CodingKeys: String, CodingKey {
case status
case vehicleList
}
init(status: String?, vehicleList: [VehicleList]?) {
self.status = status
self.vehicleList = vehicleList
}
}
class VehicleList: Codable {
let deviceImei, vehicleName, status: Int?
enum CodingKeys: String, CodingKey {
case deviceImei
case vehicleName
case status
}
init(deviceImei: String?, vehicleName: String?, status: Int?) {
self.deviceImei = deviceImei
self.vehicleName = vehicleName
self.status = status
}
}
Project structure(MVVM)
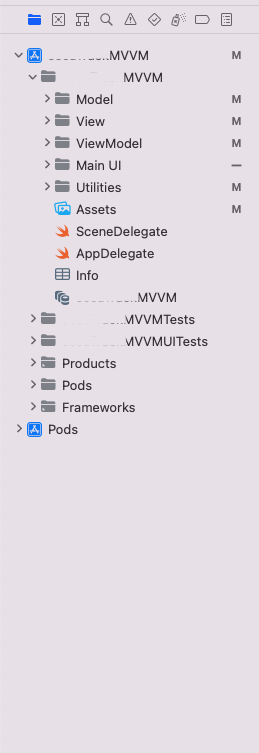
UITableViewCell-View folder mina code
//- update code as per your module data
import UIKit
class LastLocation_TableViewCell: UITableViewCell {
@IBOutlet weak var backView: UIView!
@IBOutlet weak var frontView: UIView!
@IBOutlet weak var vID: UILabel!
@IBOutlet weak var vIndentNo: UILabel!
@IBOutlet weak var vDateTime: UILabel!
@IBOutlet var vStatus: UILabel!
var modelBind: VehicleListMain?{
didSet{
userConfiguration()
}
}
func userConfiguration(){
vID.text = modelBind?.id
vIndentNo.text = modelBind?.vehicleName
vDateTime.text = modelBind?.deviceDateTime
if modelBind?.vStatus == 0 {
vRuningState = "Store"
vIndentNo.textColor = UIColor.systemRed
vStatus.text = vStatus
backView.backgroundColor = UIColor.systemRed
}
else if modelBind?.vStatus == 1 {
vRuningState = "Running"
vIndentNo.textColor = UIColor.systemGreen
backView.backgroundColor = UIColor.systemGreen
vStatus.text = vStatus
}else if modelBind?.vStatus == 2 {
vRuningState = "Stop"
vIndentNo.textColor = UIColor.systemGray
backView.backgroundColor = UIColor.systemGray
vStatus.text = vStatus
}
}
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
}
ViewModel folder
//
import Alamofire
import Foundation
import SwiftyJSON
import UIKit
import Toaster
class LastLocationViewModel{
weak var vc: ViewController?
var checkArray : NSMutableArray = NSMutableArray()
let sum = 1
let sum1 = 1
let sum2 = 1
var arrLocation = [VehicleListMain]()
var arrLocationTemp = [VehicleListMain]()
var arrLocationGreenTemp = [VehicleListMain]()
var arrLocationRedTemp = [VehicleListMain]()
var arrLocationGrayTemp = [VehicleListMain]()
var searchedArray = [VehicleListMain]()
var userName : NSMutableArray = NSMutableArray()
func getLastLocation(urlring : String , para1 : Parameters) {
ERProgressHud.show()
AF.request( urlring,method: .post, parameters: para1) .validate()
.responseString { [self]response in
switch(response.result){
case .success(let data1):
// data1 = data
if let json=data1.data(using: .utf8){
do {
let res = try JSONDecoder().decode(MyMainResponce<VehicleListMain>.self, from: json)
vTotalRedVehicleCount = 0
vTotalGreenVehicleCount = 0
vTotalGrayVehicleCount = 0
if res.status == "success"
{
for i in res.VehicleListMain{
if (i.vehicleStatus == 0) {
vTotalRedVehicleCount += sum
arrLocationRedTemp.append(i)
}
else if (i.vehicleStatus == 1)
{
vTotalGreenVehicleCount += sum1
arrLocationGreenTemp.append(i)
}
else if (i.vehicleStatus == 2)
{
vTotalGrayVehicleCount += sum2
arrLocationGrayTemp.append(i)
}
}
self.arrLocation = arrLocationGreenTemp + arrLocationRedTemp + arrLocationGrayTemp
arrLocationMain = self.arrLocation
if (getSideMenuIndex == 0)
{
DispatchQueue.main.async {
self.vc?.TableView.reloadData()
}
self.vc?.vGreenData.text = String(vTotalGreenVehicleCount)
self.vc?.vRedData.text = String(vTotalRedVehicleCount)
self.vc?.vGrayData.text = String(vTotalGrayVehicleCount)
//--for track
DispatchQueue.main.async {
self.vcTrack?.TableView.reloadData()
}
self.vcTrack?.vGreenData.text = String(vTotalGreenVehicleCount)
self.vcTrack?.vRedData.text = String(vTotalRedVehicleCount)
self.vcTrack?.vGrayData.text = String(vTotalGrayVehicleCount)
}else if getSideMenuIndex == 1
{
DispatchQueue.main.async {
self.vc2?.TableView.reloadData()
}
self.vc2?.vGreenData.text = String(vTotalGreenVehicleCount)
self.vc2?.vRedData.text = String(vTotalRedVehicleCount)
self.vc2?.vGrayData.text = String(vTotalGrayVehicleCount)
}else if getSideMenuIndex == 2
{
}
ERProgressHud.hide()
}else
{
ERProgressHud.hide()
vc?.errMsgs(Title: res.result!, MessageTxt:res.message!)
}
}
catch {
ERProgressHud.hide()
print("Error in get json datanot -")
Toast(text: "No dat found!", delay: 1, duration: 3).show()
vc?.errMsgs(Title: "Info", MessageTxt:"No data found!!")
}
}
case .failure(let err) :
/// resolver.reject(err)
ERProgressHud.hide()
vc?.errMsgs(Title: "Error", MessageTxt:"Response status code was unacceptable!")
print(err.localizedDescription)
}
}
}
func fillVehicleCount()
{
self.vc2?.vGreenData.text = String(vTotalGreenVehicleCount)
self.vc2?.vRedData.text = String(vTotalRedVehicleCount)
self.vc2?.vGrayData.text = String(vTotalGrayVehicleCount)
}
func _refillTrackList()
{
self.vcTrack?.vGreenData.text = String(vTotalGreenVehicleCount)
self.vcTrack?.vRedData.text = String(vTotalRedVehicleCount)
self.vcTrack?.vGrayData.text = String(vTotalGrayVehicleCount)
}
}
extension ViewController: UITableViewDataSource{
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
if searching {
return viewModeLoc.searchedArray.count
} else {
return viewModeLoc.arrLocation.count
}
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as! LastLocation_TableViewCell
if searching {
cell.modelBind = viewModeLoc.searchedArray[indexPath.row]
}else
{
cell.modelBind = viewModeLoc.arrLocation[indexPath.row]
}
return cell
}
}
final output with MVVM pattern
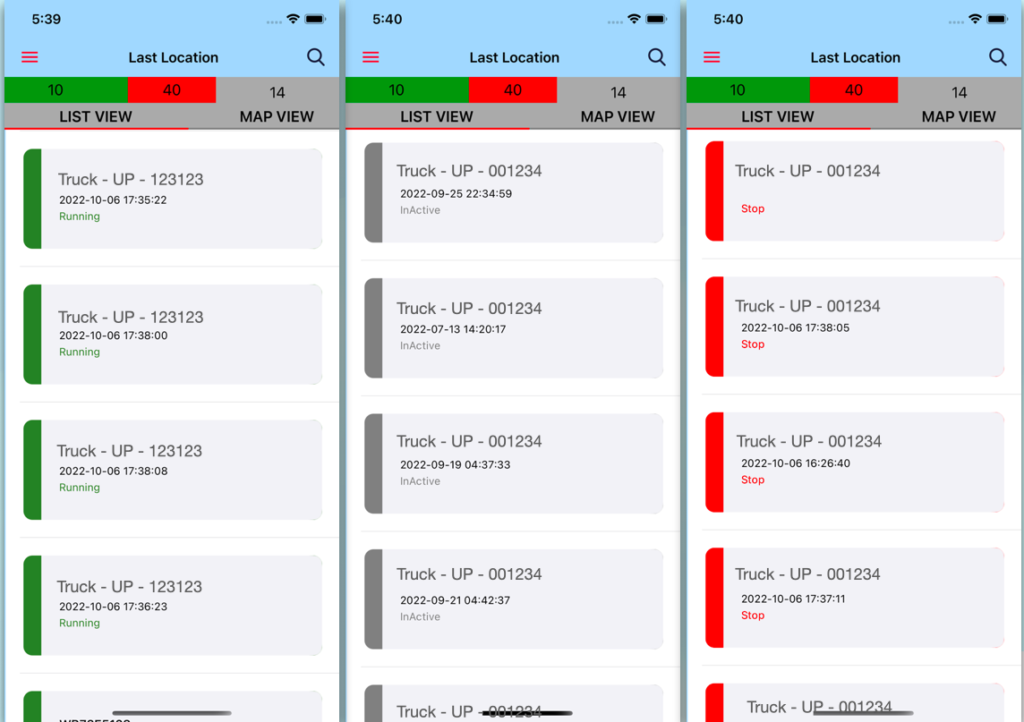
I hope it was a helpful article, please share and subscribe to my channel, Thanks for reading and if you have any questions or comments, See you soon.