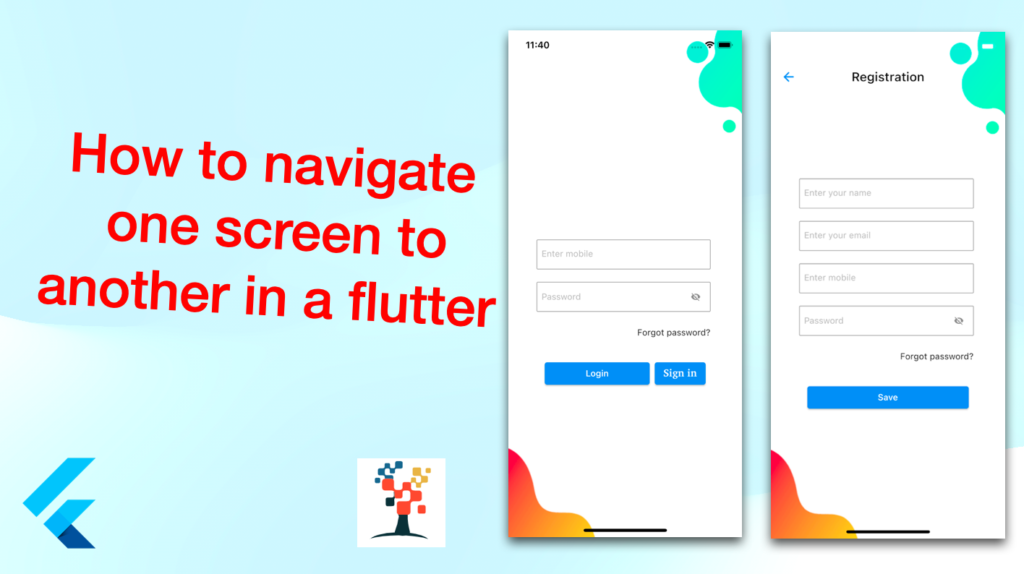
How to navigate from one screen to another in a flutter.
Every day we have expended the most time on mobile apps to learn new things, web searches, social media, and much more used we notice the click from one screen to another screen jump in apps and the web so how can it possible the relationship between one screen to another screen in flutter app.
The route framework is one of the most important in any mobile application improvement structure. In this, the user can connect one screen to another screen page.
So, in this article, we will see how to navigate to a new screen and from one screen to another in a flutter.
Navigate between screens in Flutter
In this article, we have used two screens, they are log-in and registration which extends StatefulWidget. They both have different state classes and widgets.
In Login, we shall place a two-button, two-text-form-field like user name, password, and forget password label below the password text-form-field, when this login button is pressed, we shall navigate to the second screen, Registration. In Registration, we shall place a RaisedButton top arrow and when this button is pressed, we shall navigate back to the first screen Login screen. The fully running code is available below.
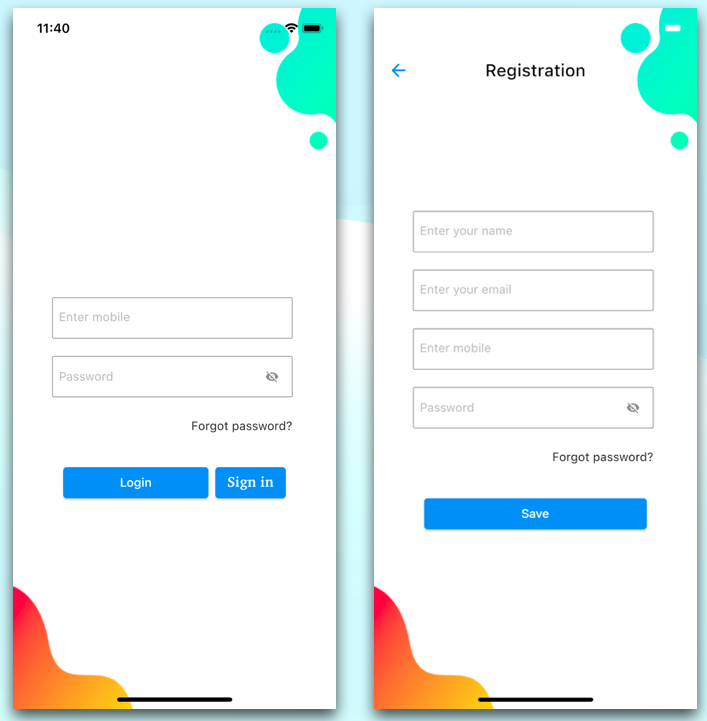
Use Navigation. push with MaterialPageRoute
Navigator.push(context,MaterialPageRoute(builder: (context) => regForm()));
Using the Sprinkle library function
context.display(regForm());
Navigation back
Navigator.pop(context);
Using the Sprinkle library function
context.back();
Using the remove all back screen
Navigator.pushAndRemoveUntil(
context,
MaterialPageRoute(
builder: (context) => Your_controller_Form()),
ModalRoute.withName("/Your_controller_Form"));
App bar Example

Login screen
import 'package:flutter/material.dart';
import 'package:flutter_svg/flutter_svg.dart';
import 'package:securisk_app/other_desgin/screens/choose_language.dart';
import 'package:securisk_app/other_desgin/screens/google_map.dart';
import 'package:securisk_app/other_desgin/screens/login_and_reg/forget_pass.dart';
import 'package:securisk_app/other_desgin/screens/login_and_reg/google_map2.dart';
import 'package:securisk_app/other_desgin/screens/login_and_reg/map.dart';
import 'package:securisk_app/other_desgin/screens/reg_form.dart';
import 'package:securisk_app/utlity/colors.dart';
import 'package:securisk_app/widgets/bg_image.dart';
class loginMain extends StatefulWidget {
@override
State<loginMain> createState() => _loginMainState();
}
class _loginMainState extends State<loginMain> {
final emailController = TextEditingController();
final passController = TextEditingController();
bool isShowPass = false;
bool onError = false;
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/images/bg.png"),
fit: BoxFit.cover,
),
),
child: Scaffold(
backgroundColor: Colors.transparent,
body: Container(
padding: const EdgeInsets.symmetric(horizontal: 15, vertical: 10),
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const SizedBox(
height: 15,
),
const SizedBox(
height: 200,
),
// ignore: prefer_const_constructors
Padding(
padding: const EdgeInsets.only(left: 30.0),
child: const Text('E-mail'),
),
Container(
padding: const EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
style: const TextStyle(color: Colors.black, fontSize: 14),
controller: emailController,
decoration: InputDecoration(
prefixIcon: const Icon(Icons.email_outlined),
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: const EdgeInsets.fromLTRB(8, 5, 10, 5),
labelText: "Your email",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle:
TextStyle(color: Colors.grey.shade400, fontSize: 14),
),
// inputFormatters: [new LengthLimitingTextInputFormatter(10)],
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? const Positioned(
bottom: 0,
child: Padding(
padding: EdgeInsets.only(left: 30),
child: Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 30.0),
child: Text('Password'),
),
Container(
padding: EdgeInsets.only(left: 30, right: 35, top: 10),
child: TextFormField(
controller: passController,
obscureText: isShowPass,
decoration: InputDecoration(
prefixIcon: Icon(Icons.lock),
labelText: "Your Password",
alignLabelWithHint: true,
floatingLabelBehavior: FloatingLabelBehavior.never,
contentPadding: EdgeInsets.fromLTRB(8, 5, 10, 5),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(2.0),
),
labelStyle:
TextStyle(color: Colors.grey.shade400, fontSize: 14),
suffixIcon: GestureDetector(
onTap: () {
setState(() {
isShowPass = !isShowPass;
});
},
child: Icon(
isShowPass
? Icons.visibility
: Icons.visibility_off,
size: 16,
)),
),
validator: (String? val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = RegExp(patttern);
if (val!.isEmpty) {
return "Mobile is Required";
} else if (val.length != 10) {
return "Mobile number must 10 digits";
} else if (!regExp.hasMatch(val)) {
return "Mobile Number must be digits";
}
return null;
},
)),
onError
? Positioned(
bottom: 0,
child: Padding(
padding: const EdgeInsets.only(left: 30),
child: Text('', style: TextStyle(color: Colors.red)),
))
: Container(),
SizedBox(
height: 10,
),
GestureDetector(
onTap: () => Navigator.push(context,
MaterialPageRoute(builder: (context) => ForgetPassword())),
child: Align(
alignment: Alignment.topRight,
child: Padding(
padding: const EdgeInsets.only(right: 35.0),
child: Text(
"Forgot password?",
style: TextStyle(color: AppColors.blueColor),
),
),
),
),
SizedBox(
height: 25,
),
Padding(
padding: EdgeInsets.only(left: 35, right: 35),
child: Container(
padding: EdgeInsets.all(8.0),
// height: 100,
child: Row(
children: [
Expanded(
child: Container(
//height: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppColors.buttonColor,
onPrimary: Colors.white,
side: BorderSide(
color: AppColors.buttonColor, width: 5),
),
onPressed: () async {
Navigator.pushAndRemoveUntil(
context,
MaterialPageRoute(
builder: (context) => ChooseLanguage()),
ModalRoute.withName("/chooseLaguage"));
},
child: Text("Login"),
),
)),
Container(
padding: EdgeInsets.only(left: 8.0),
//height: 100,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppColors.buttonColor,
onPrimary: Colors.white,
side: BorderSide(
color: AppColors.buttonColor, width: 5),
),
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => regForm()));
},
child: Text("SingUp")))
],
),
),
),
Spacer(),
SizedBox(
height: 10,
),
],
),
),
),
);
}
}
How to navigate one screen to another in a flutter
I hope it was a helpful article, please share and subscribe to my channel, Thanks for reading and if you have any questions or comments, See you soon in the next article.