In Flutter, the DateTime
class is used to represent a specific point in time, with a precision of microseconds. The class has several constructors that can be used to create a new instance of the class, including now()
, which creates a new instance representing the current date and time,
and fromMillisecondsSinceEpoch(int millisecondsSinceEpoch)
,
which creates a new instance representing the specified number of milliseconds since the epoch.
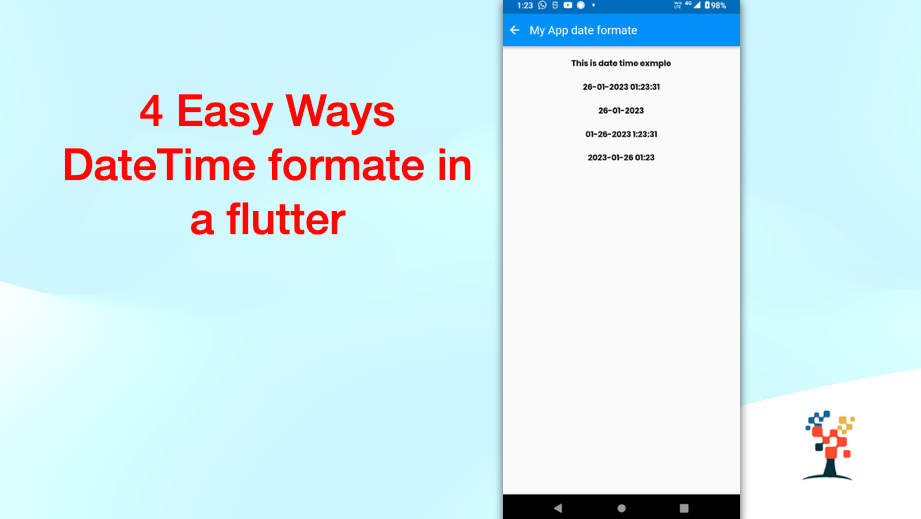
DateTime
The DateTime class has several properties that can be used to access the different components of the date and time, such as year, month, day, hour, minute, and second. You can also use the isBefore, isAfter, and isAtSameMomentAs methods to compare two DateTime objects.
DateTime Class
In addition to the DateTime class, the Duration class can be used to represent a duration of time. This can be used to perform calculations involving dates and times, such as adding or subtracting a duration from a DateTime object.
To display dates and times in a user-friendly format, you can use the DateFormat class from the intl package. The package can be added to your project by adding the following line to your pubspec.yaml file:
dependencies:
intl: ^0.16.1
Date Formate
You can then import the package and create a new instance of the DateFormat class and specify the format you want to use. For example, to display the date in the format “dd/MM/yyyy”, you can use the following code:
DateFormat dateFormat = new DateFormat("dd/MM/yyyy");
DateTime now = new DateTime.now();
print(dateFormat.format(now));
Time Formate
To display the time in the format “HH:mm:ss”, you can use the following code:
DateFormat timeFormat = new DateFormat("HH:mm:ss");
print(timeFormat.format(now));
String Formate (parse metode)
You can also use the parse method of the DateFormat class to parse a string representation of a date or time and convert it to a DateTime object. For example, to parse a string in the format “dd/MM/yyyy” and convert it to a DateTime object with date range format, you can use the following code:
String dateString = "01/01/2021";
DateTime date = dateFormat.parse(dateString);
print(date);
There are many other methods and properties available in the DateFormat class, such as locale, pattern, and add which can be used to customize the format of the date and time as per your requirement.
It’s also worth mentioning that flutter also provides a built-in Text widget that can be used to display dates and times in a user-friendly format. The Text widget has a property called data which can be used to display the date and time.
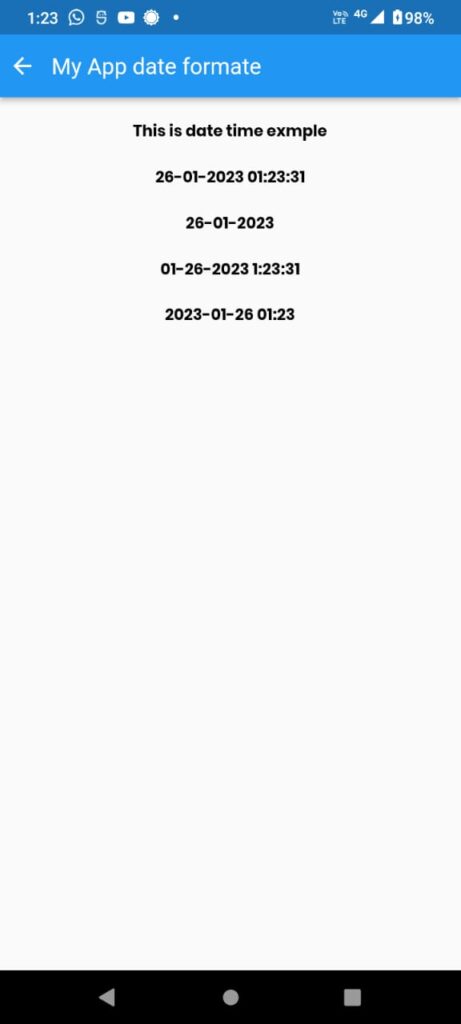
Final code
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import 'package:google_fonts/google_fonts.dart';
class Exmaple extends StatefulWidget {
@override
State<Exmaple> createState() => _ExmapleState();
}
class _ExmapleState extends State<Exmaple> {
String? date1, date2, date3, date4;
@override
void initState() {
myDate();
super.initState();
}
void myDate() {
var now = DateTime.now();
var formatter = DateFormat('dd-MM-yyyy');
String formattedDate = formatter.format(now);
var now1 = DateTime.now();
var formatter1 = DateFormat('dd-MM-yyyy HH:mm:ss');
String formattedDate1 = formatter1.format(now1);
var now3 = DateTime.now();
var formatter3 = DateFormat('MM-dd-yyyy H:m:s');
String formattedDate3 = formatter3.format(now3);
var now4 = DateTime.now();
var formatter4 = DateFormat('yyyy-MM-dd kk:mm');
String formattedDate4 = formatter4.format(now4);
date1 = formattedDate1;
date2 = formattedDate;
date3 = formattedDate3;
date4 = formattedDate4;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('My App date formate'),
centerTitle: false,
automaticallyImplyLeading: false,
leadingWidth: 30,
leading: IconButton(
icon: const Icon(Icons.arrow_back, color: Colors.white),
onPressed: () => Navigator.of(context).pop(),
),
),
body:
Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.start,
children: [
SizedBox(
height: 20,
),
Center(
child: Text(
"This is date time exmple",
style: TextStyle(
color: Colors.black,
fontFamily: 'Poppins',
fontWeight: FontWeight.w800,
),
),
),
SizedBox(
height: 20,
),
Center(
child: Text(
date1.toString(),
style: TextStyle(
color: Colors.black,
fontFamily: 'Poppins',
fontWeight: FontWeight.w800,
),
),
),
SizedBox(
height: 20,
),
Center(
child: Text(
date2.toString(),
style: TextStyle(
color: Colors.black,
fontFamily: 'Poppins',
fontWeight: FontWeight.w800,
),
),
),
SizedBox(
height: 20,
),
Center(
child: Text(
date3.toString(),
style: TextStyle(
color: Colors.black,
fontFamily: 'Poppins',
fontWeight: FontWeight.w800,
),
),
),
SizedBox(
height: 20,
),
Center(
child: Text(
date4.toString(),
style: TextStyle(
color: Colors.black,
fontFamily: 'Poppins',
fontWeight: FontWeight.w800,
),
),
),
],
),
);
}
}
Conclusion
The DateTime and Duration classes can be used to represent and manipulate dates and times in Flutter, while the DateFormat class from the intl package can be used to format dates and times in a user-friendly way. It is also possible to use the built-in Text widget to display the date and time.