In this article, I have discussed a very useful topic In every mobile app development process needs this topic, How to upload a profile image in a flutter, To upload a profile image in a Flutter REST API, you can use the HTTP package to send a multipart/form-data request to the API endpoint. Here’s an example of how to do it.
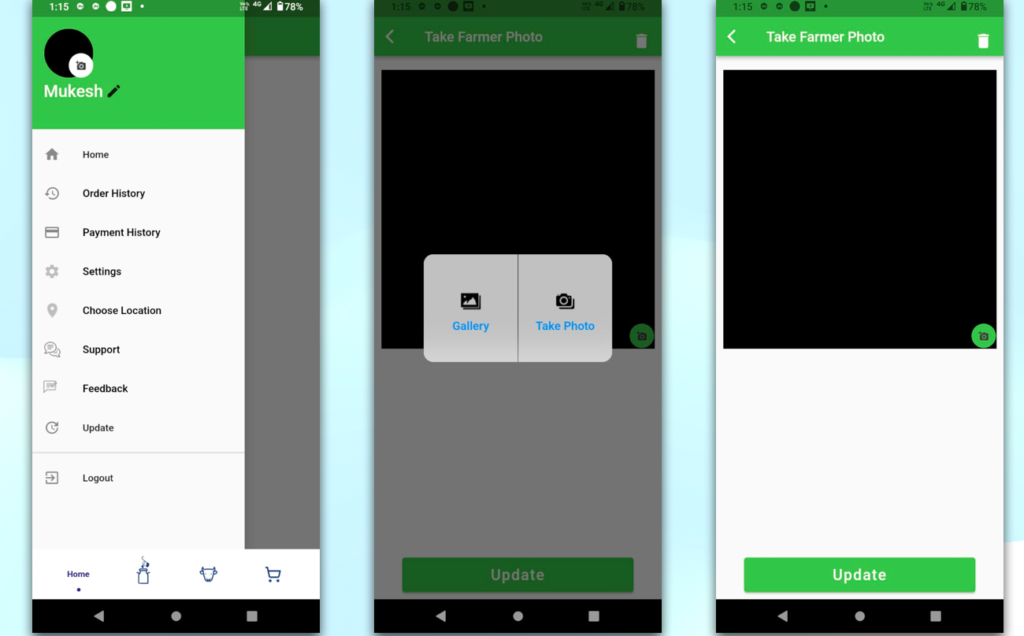
if you need the setup in windows/mac OS in flutter so please go through this link.
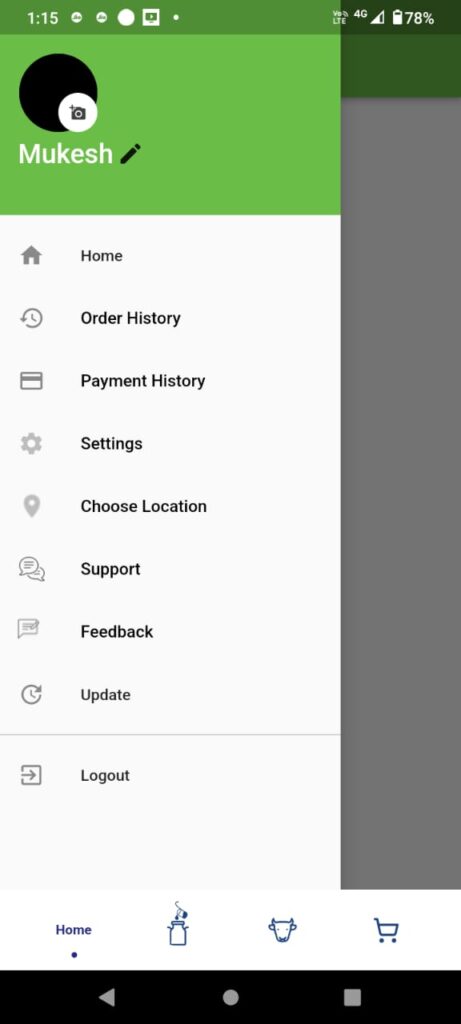
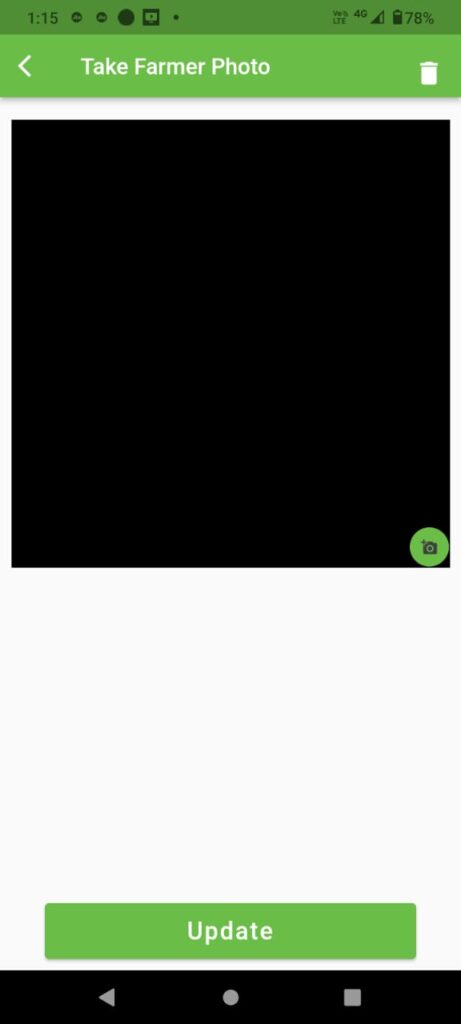
Let’s Start
This article is cover the below topic in flutter app development
- How to upload images to the server I flutter?
- How to upload images and display them on flutter?
- How to upload an Image using multipart in Flutter?
- Flutter upload image, save in server?
- Flutter upload image in REST API?
- Flutter app upload image?
- Save profile image in flutter?
- Get the image in URL in flutter?
- How to upload profile images in REST API?
- Flutter upload image?
- Capturing Photos Using the Camera in Flutter
How to fetch data from REST API
How to display the side menu profile image
This screen shows the drawer as per the user’s need changes the design has two major things on below.
1- profile image desplay .
2- profile image clickable and show the icon
3- variable declaration for holding the image URL and showing the Image.
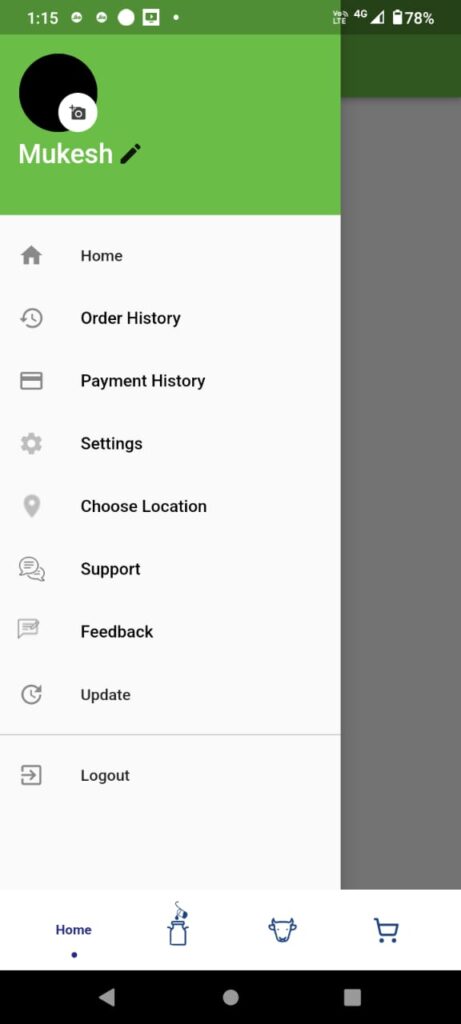
How to upload images and display them on flutter
//------------user image and other deatils //
Container(
child: UserAccountsDrawerHeader(
decoration: BoxDecoration(
color: WidgetColors.mainHeaderColor,
),
accountName: InkWell(
onTap: () {
},
child: Text.rich(
TextSpan(
children: [
TextSpan(
text: myAccountName ?? 'NA',
style: TextStyle(
color: Colors.white,
fontSize: 24.0,
),
),
TextSpan(text: ' '),
],
),
),
),
accountEmail: Text(myGroupType),
currentAccountPicture: InkWell(
onTap: () {
//edit image link click as per your need.
},
child: Stack(
children: <Widget>[
Container(
width: 250,
height: 250,
child: CircleAvatar(
backgroundColor: AppTheme.mainColor,
radius: 43,
child: ClipOval(
child: FadeInImage(
fadeInDuration: const Duration(milliseconds: 500),
placeholder:
const AssetImage('assets/images/userL.png'),
image: vThumbImage == null
? NetworkImage(
"https://img.icons8.com/fluency/48/null/no-image.png")
: NetworkImage(
vThumbImage!),
imageErrorBuilder: (context, error, stackTrace) {
return Container(
child: Image.asset("assets/images/userL.png"));
},
fit: BoxFit.cover, height: 70, width: 70,
),
),
),
),
Positioned(
bottom: 1,
right: 1,
child: Container(
height: 35,
width: 35,
child: InkWell(
// onTap: _onAlertPress,
child: const Icon(
Icons.add_a_photo,
size: 15.0,
color: Color(0xFF404040),
),
),
decoration: const BoxDecoration(
color: Colors.white,
borderRadius:
BorderRadius.all(Radius.circular(20))),
))
],
),
),
)),
//--------menu list ------------------//
Packag – image_picker
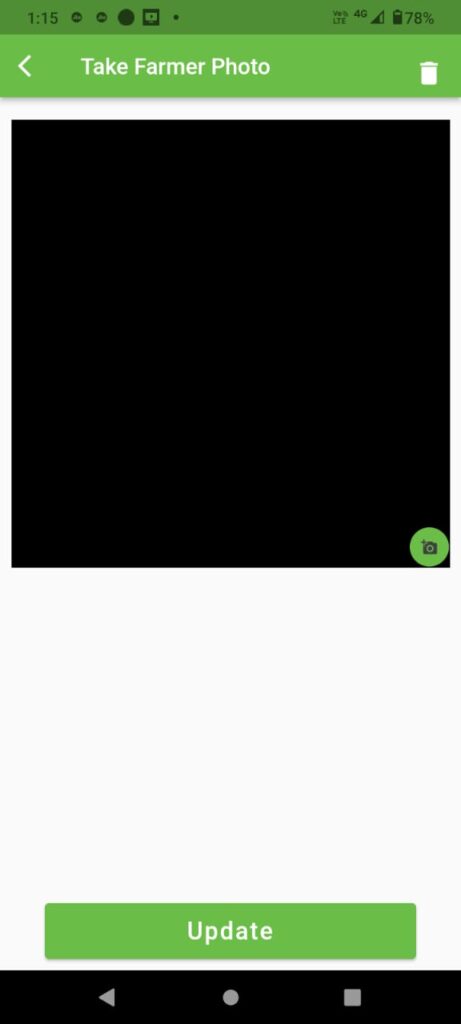
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:image_picker/image_picker.dart';
import 'dart:convert';
import 'dart:io';
import 'package:progress_dialog_null_safe/progress_dialog_null_safe.dart';
import 'package:http/http.dart' as http;
import 'package:shared_preferences/shared_preferences.dart';
ProgressDialog? pr;
final ImagePicker _picker = ImagePicker();
bool oldImage = true;
bool newImage = false;
File? imageURI;
bool isLoding = false;
var name = TextEditingController();
final _formKey = GlobalKey<FormState>();
Future<void> getImage() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
vImageId = prefs.getString('PhotoId') ?? '';
}
Image Display Box(Zoom)
Widget oldImageWidget() {
return Visibility(
visible: oldImage,
child: Container(
width: 400,
height: 400,
child: FadeInImage(
fadeInDuration: const Duration(milliseconds: 500),
placeholder: const AssetImage('assets/images/userL.png'),
image: vThumbImage == null
? NetworkImage(
"https://xxx/no-image.png")
: NetworkImage(
vThumbImage!),
imageErrorBuilder: (context, error, stackTrace) {
return Container(child: Image.asset("assets/images/userL.png"));
},
fit: BoxFit.cover, height: 400,
width: 400,
),
),
);
}
Widget newImageChoose() {
return Container(
width: 400,
height: 400,
child: Visibility(
visible: newImage,
child: Container(
width: 400,
height: 400,
child: imageURI == null
? const Text(
'No Image',
)
: Image.file(
imageURI!,
height: 400,
width: 400,
fit: BoxFit.cover,
),
),
),
);
}
Main Widget (REST API Integration)
@override
Widget build(BuildContext context) {
//============================================= loading dialoge
pr = new ProgressDialog(context, type: ProgressDialogType.normal);
//Optional
pr!.style(
message: 'Please wait...',
borderRadius: 10.0,
backgroundColor: Colors.white,
progressWidget: CircularProgressIndicator(),
elevation: 10.0,
insetAnimCurve: Curves.easeInOut,
progressTextStyle: const TextStyle(
color: Colors.black, fontSize: 13.0, fontWeight: FontWeight.w400),
messageTextStyle: const TextStyle(
color: Colors.black, fontSize: 19.0, fontWeight: FontWeight.w600),
);
return Scaffold(
appBar: AppBar(
backgroundColor: AppTheme.mainColor,
leading: IconButton(
icon: const Icon(Icons.arrow_back_ios),
onPressed: () {
Navigator.pop(context);
},
),
title: const Text('Take Farmer Photo'),
actions: [],
),
body: Container(
child: Container(
//color: Colors.white,
child: Padding(
padding: const EdgeInsets.only(
right: 10,
left: 10,
),
child: SingleChildScrollView(
child: Column(
children: <Widget>[
Container(
margin: const EdgeInsets.only(top: 20),
alignment: Alignment.center,
child: Column(
children: <Widget>[
Container(
decoration: const BoxDecoration(
color: WidgetColors.mainHeaderColor,
borderRadius:
BorderRadius.all(Radius.circular(20))),
child: Stack(children: [
Stack(
children: <Widget>[
Visibility(
visible: oldImage,
child: Container(
width: 400,
height: 400,
child: oldImageWidget(),
),
),
Visibility(
visible: newImage,
child: Container(
width: 400,
height: 400,
child: newImageChoose(),
),
),
Positioned(
bottom: 1,
right: 1,
child: Container(
height: 35,
width: 35,
child: InkWell(
onTap: _onClickPress,
child: const Icon(
Icons.add_a_photo,
size: 15.0,
color: Color(0xFF404040),
),
),
decoration: const BoxDecoration(
color: WidgetColors.mainHeaderColor,
borderRadius: BorderRadius.all(
Radius.circular(20))),
))
],
),
]),
)
],
),
),
Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Visibility(
visible: false,
child: TextFormField(
onChanged: (v) => setState(() {
vAccountName = v;
}),
initialValue: vAccountName,
onSaved: (val) => vAccountName = val!,
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: 'Name',
hintText: 'Enter your name',
// icon: Icon(Icons.person),
isDense: true,
),
),
),
],
),
),
],
),
),
),
),
),
bottomNavigationBar: Container(
height: 50,
margin: EdgeInsets.only(left: 40, top: 10, right: 40, bottom: 10),
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppTheme.mainColor, elevation: 2),
onPressed: () async {
if (_formKey.currentState!.validate()) {
if (imageURI == null) {
Fluttertoast.showToast(
msg: "Please Select Image",
toastLength: Toast.LENGTH_LONG,
gravity: ToastGravity.CENTER,
timeInSecForIosWeb: 1,
backgroundColor: WidgetColors.mainTextColor,
textColor: Colors.white,
fontSize: 16.0);
} else {
pr!.show();
var res = await uploadImageNew(imageURI!.path);
setState(() {
if (res == 'success') {
pr!.hide();
print('Photo uplode successfully');
Navigator.pushAndRemoveUntil(
context,
MaterialPageRoute(
builder: (context) => const HomePage()),
ModalRoute.withName("/home"));
} else {
pr!.hide();
Fluttertoast.showToast(
msg: "Image Not Uploaded",
toastLength: Toast.LENGTH_LONG,
gravity: ToastGravity.CENTER,
timeInSecForIosWeb: 1,
backgroundColor: Colors.red,
textColor: Colors.white,
fontSize: 16.0);
}
});
}
}
},
child: Center(
child: Text(
"Update",
style: TextStyle(
color: Colors.white,
letterSpacing: 1.5,
fontSize: MediaQuery.of(context).size.height / 40,
),
),
),
),
),
);
}
Capturing Photos Using the Camera in Flutter
// ================================= Image from camera ======
List<XFile>? _imageFileList;
Future getCameraImage() async {
XFile? image = await _picker.pickImage(source: ImageSource.camera);
final File? imagefile = File(image!.path);
Image.file(imagefile!);
File file = File(imagefile.path);
setState(() {
imageURI = imagefile;
oldImage = false;
newImage = true;
imageURI == null
? const Text(
'No image',
style: TextStyle(fontSize: 10),
)
: Image.file(imagefile, fit: BoxFit.cover);
Navigator.pop(context);
});
}
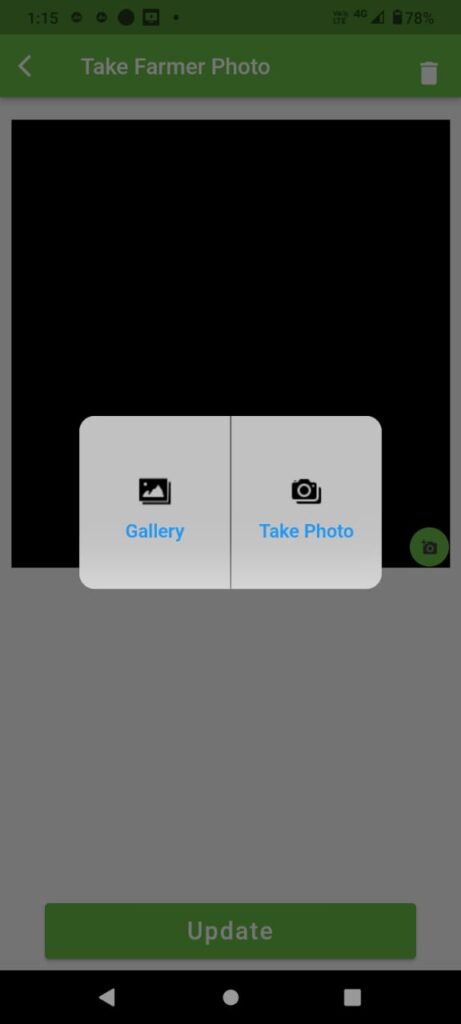
Pick an Image from the Gallery in Flutter
//============================== Image from gallery
Future getGalleryImage() async {
XFile? image = await _picker.pickImage(
source: ImageSource.gallery,
imageQuality: 25,
);
final File? imagefile = File(image!.path);
Image.file(imagefile!);
File file = File(imagefile.path);
setState(() {
imageURI = imagefile;
oldImage = false;
newImage = true;
imageURI == null
? const Text(
'No image',
style: TextStyle(fontSize: 8),
)
: Image.file(imagefile, fit: BoxFit.cover);
Navigator.pop(context);
});
}
Image Upload Function in REST API(MultipartFile)
Future<String> uploadImageNew(filename) async {
isLoding = true;
print(vBaseUrl + ApiConstants.apiProfilePhototEdit);
var request = http.MultipartRequest(
'POST', Uri.parse(vBaseUrl + ApiConstants.apiProfilePhototEdit));
request.fields['name'] = vAccountName ?? 'NA';
request.fields['AccessToken'] = vAccessToken;
request.files.add(await http.MultipartFile.fromPath('photo', filename));
var res = await request.send();
if (200 == res.statusCode) {
isLoding = false;
String body = await res.stream.bytesToString();
var jsonString = res.reasonPhrase;
var jsonMap = json.decode(body);
var myOTPRespo = ImageReturnRespo.fromJson(jsonMap);
SharedPreferences pref = await SharedPreferences.getInstance();
vAccountName = myOTPRespo.name.toString();
pref.setString('vName', myOTPRespo.name.toString());
if (myOTPRespo.images!.isEmpty || myOTPRespo.images == null) {
print('image blank');
vThumbImage = 'https://yourImagesURL/no-image.png';
pref.setString('PhotoId', 'Not_Available');
pref.setString('PhotoshowStatus', '0');
pref.setString('FarmerImage',
'https://yourImagesURL/no-image.png');
} else {
print('image avialabe');
if (myOTPRespo.images![0].isDefault == '1') {
vThumbImage = myOTPRespo.images![0].imagePath ??
'https://yourImagesURL/no-image.png';
pref.setString(
'FarmerImage', myOTPRespo.images![0].imagePath.toString());
pref.setString('PhotoId', myOTPRespo.images![0].id.toString());
pref.setString(
'PhotoshowStatus', myOTPRespo.images![0].isDefault.toString());
} else {
vThumbImage = 'https://yourImagesURL/no-image.png';
pref.setString('PhotoId', 'Not_Available');
pref.setString('PhotoshowStatus', '0');
pref.setString('FarmerImage',
'https://yourImagesURL/no-image.png');
}
}
var otpStatus = myOTPRespo.status.toString();
if (otpStatus == "success") {
Fluttertoast.showToast(
msg: myOTPRespo.message.toString(),
toastLength: Toast.LENGTH_SHORT,
gravity: ToastGravity.BOTTOM,
timeInSecForIosWeb: 1,
backgroundColor: Colors.green,
textColor: Colors.white,
fontSize: 16.0);
} else {
Fluttertoast.showToast(
msg: myOTPRespo.message.toString(),
toastLength: Toast.LENGTH_SHORT,
gravity: ToastGravity.BOTTOM,
timeInSecForIosWeb: 1,
backgroundColor: Colors.red,
textColor: Colors.white,
fontSize: 16.0);
}
return myOTPRespo.status.toString();
} else {
return '';
}
}
I sincerely hope that was helpful; if so, kindly share it and subscribe to my channel. Thank you for reading this article, and I’ll see you soon if you have any questions or comments please comment.