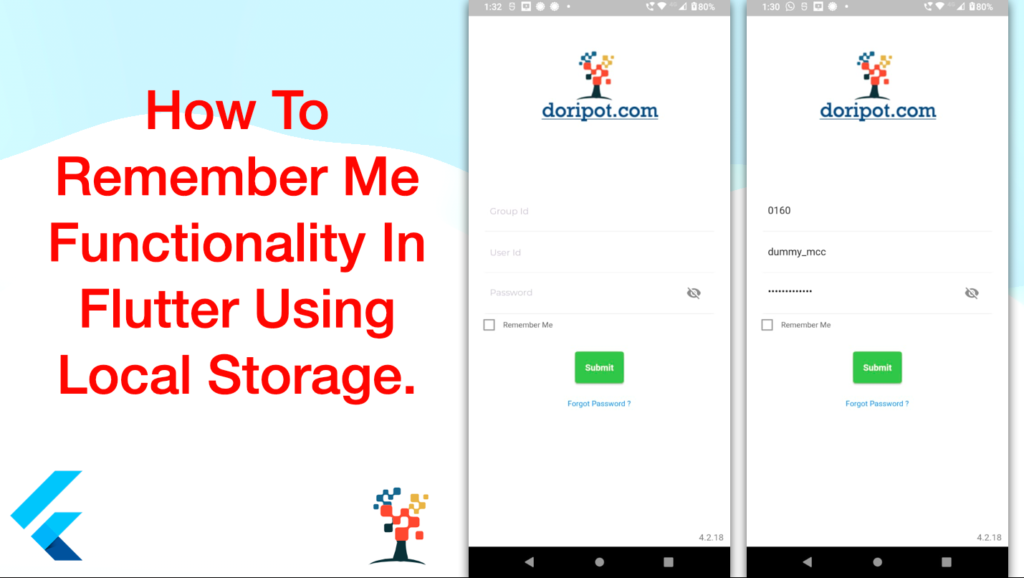
Hello Developers, welcome to our blog post about implementing Remember Me functionality in Flutter using local storage. Everyone wants to make their user experience as convenient as possible and implementing a Remember Me feature is one way to do this.
We will be looking at what the Remember Me feature is, how to integrate local storage with it, and the advantages of using this feature.
We will also look at how to implement Remember Me in Flutter. So, if you are interested in making your user experience smoother, then this post is for you!
What is Remember Me Functionality?
Remember Me functionality is a feature that allows users to store their login information so they don’t have to re-enter it every time they want to access an app or website.
It is often implemented using a combination of technologies such as Flutter and SQLite.
Flutter is a mobile development platform that helps developers create native applications for Android and iOS while SQLite is a lightweight database system that helps store user information. With the Remember Me functionality, when a user logs in, their information is securely stored so that they can automatically log in the next time they access the app or website, saving them time and effort.
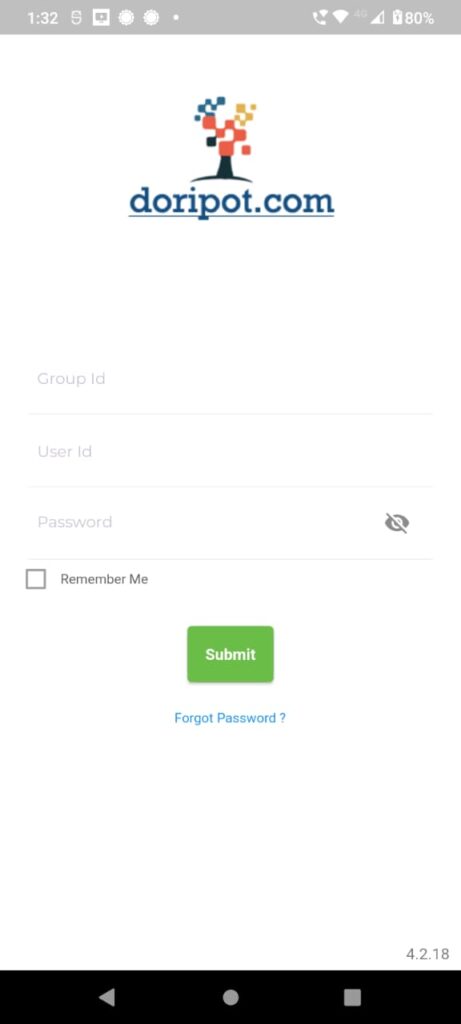
Implementing Remember Me in Flutter
If you would like to incorporate a “Remember Me” feature in your Flutter app, then you’re in luck! With the help of SQLite, it’s easy to implement this feature in a secure and straightforward way.
SQLite is a powerful library that allows you to store and retrieve data quickly, making it the perfect choice for this kind of task.
Additionally, Flutter provides a variety of tools to help you build your application in a way that makes sense to your users.
With these tools and a bit of coding knowledge, you can have a fully functioning “Remember Me” feature in your Flutter app in no time!
In this article, we use SharedPreference to save data in the local database. You have to add a shared preferences plugin in your project inside the pubspec.yml file
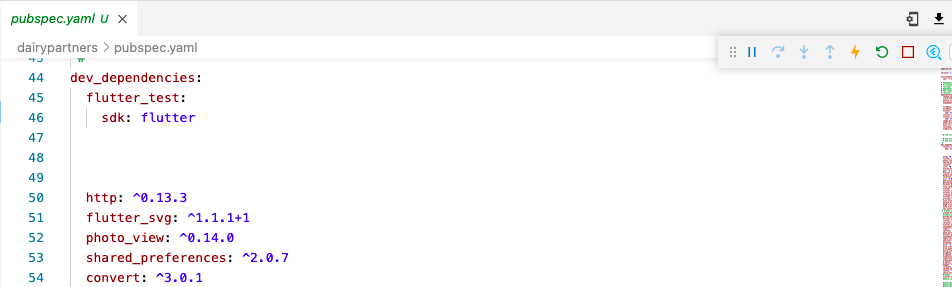
You have to add a shared preferences plugin in your project inside the pubspec.yml file
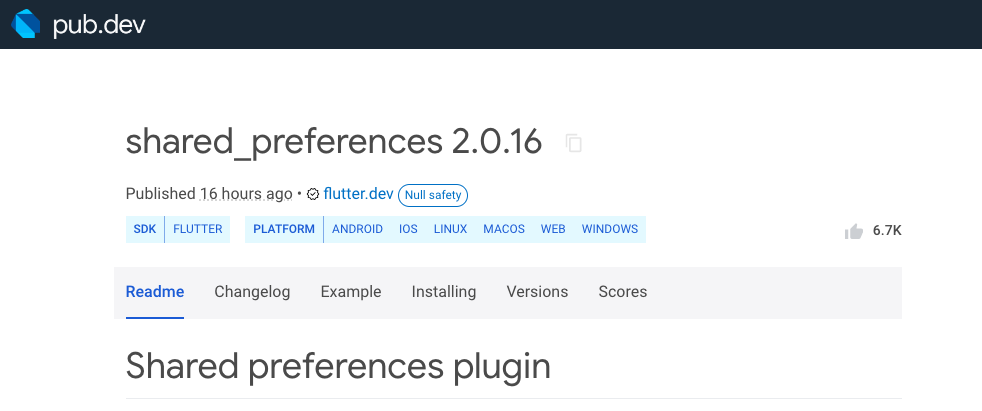
Shared preferences, Wraps platform-specific persistent storage for simple data (NSUserDefaults on iOS and macOS, SharedPreferences on Android, etc.).
Data may be persisted to disk asynchronously, and there is no guarantee that writes will be persisted to disk after returning, so this plugin must not be used for storing critical data.
Supported data types are int, double, bool, String, and List<String>.
import 'package:shared_preferences/shared_preferences.dart';
Understanding Local Storage in Flutter
Do you need assistance comprehending how to apply local storage in Flutter? Local storage is an important part of any app, and Flutter makes it easy to utilize.
With Flutter, you can use a variety of different databases, such as SQLite. SQLite is a lightweight and fast database that allows you to store data locally on the device.
With SQLite, you can store data in a structured way, such as creating fields for each type of data you want to store.
Variable initialize
bool isCheckedRememberMe = false;
It’s easy to set up, and you can access the data quickly and efficiently. Local storage in Flutter makes it easy to store data on the device and makes your app more powerful and efficient.
Remember Me? Function
actionRemeberMe(bool value) {
isCheckedRememberMe = value;
SharedPreferences.getInstance().then(
(prefs) {
prefs.setBool("remember_me", value);
prefs.setString('groupId', groupIdController.text);
prefs.setString('userId', userIdController.text);
prefs.setString('password', passwordController.text);
},
);
setState(() {
isCheckedRememberMe = value;
});
}
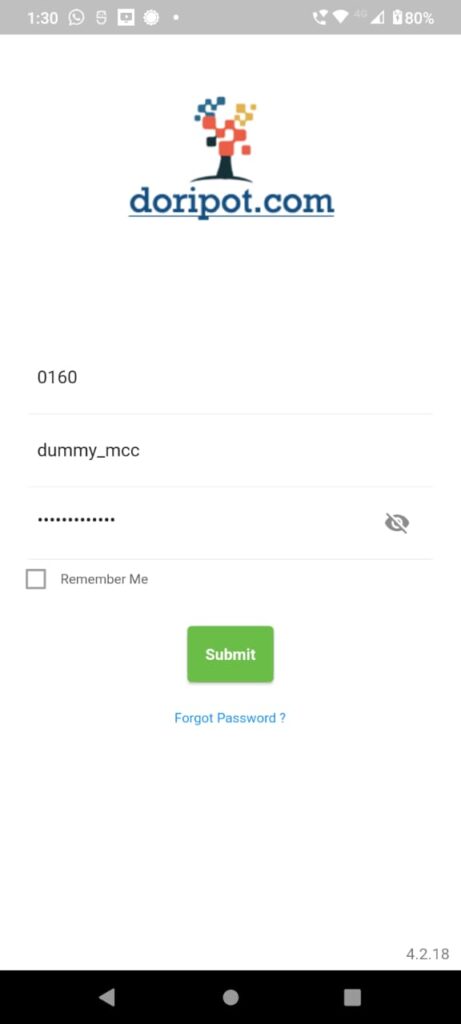
Integrating Local Storage and Remember Me Functionality
We store user data locally and providing a “Remember Me” functionality is essential for any application.
Thankfully, Flutter makes it easy to integrate local storage and remember me functionality into your project.
By leveraging the power of SQLite and Flutter’s rich set of libraries, you can quickly and easily store user data locally and provide a “Remember Me” functionality.
This will ensure your users have a consistent experience every time they open your application.
@override
void initState() {
getCheckRememberStatus();
lngBox();
getdata();
super.initState();
}
Advantages of Using Remember Me in Flutter
Using the “Remember Me” feature in your Flutter app is a great way to improve user experience and make it easier for them to sign in and use the app seamlessly.
With this feature, users don’t have to enter their login details each time they open the app. Instead, their details are securely stored in an SQLite database, which can be used to automatically log them in the next time.
This makes it easier for users to access the app without having to remember their login details. Additionally, this feature also makes the app more secure by offering an extra layer of authentication.
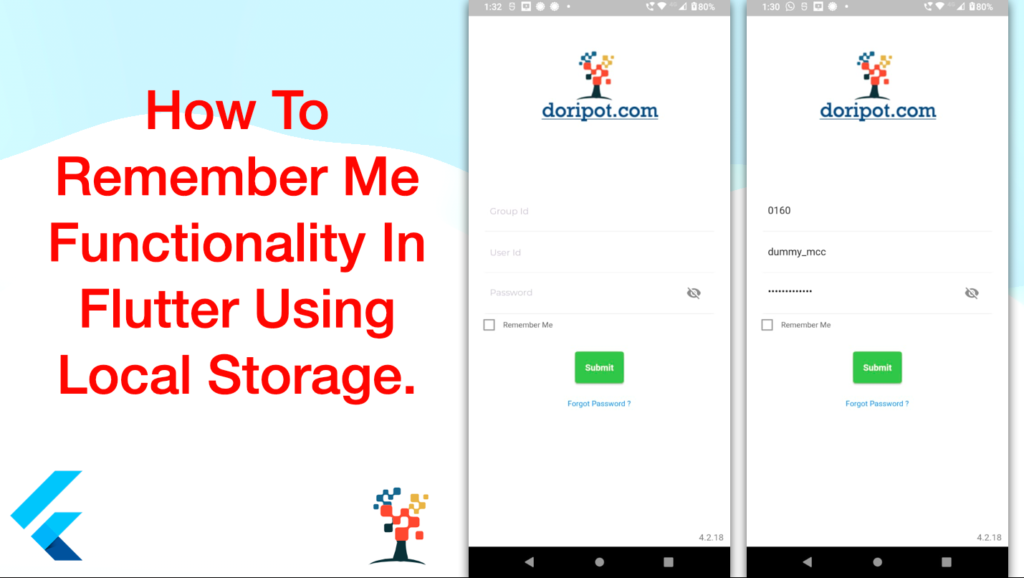
Final Code
@override
Widget build(BuildContext context) {
prg = ProgressDialog(context, type: ProgressDialogType.normal);
prg.style(
message: 'Please wait.......',
borderRadius: 3.0,
backgroundColor: Colors.grey,
progressWidget: const CircularProgressIndicator(),
maxProgress: 1.0,
);
final bloc = Provider.of<LoginBloc>(context, listen: false);
return Scaffold(
backgroundColor: Colors.white,
key: ValidationData.scaffoldKeyMobileScreen,
body: Container(
child: SingleChildScrollView(
child: Form(
key: ValidationData.formKeyValidateAddRequestScreen,
child: Container(
child: Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.only(left: 50.0, right: 50.0),
child: Container(
height: 280,
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage(
'assets/images/logo_doripot.com.png'),
fit: BoxFit.scaleDown)),
// child: Stack(
// children: <Widget>[
// ],
// ),
),
),
Padding(
padding: const EdgeInsets.all(20.0),
child: Column(
children: <Widget>[
// FadeAnimation(
// 1.8,
Container(
padding: const EdgeInsets.all(5),
child: Column(
children: <Widget>[
Container(
padding: const EdgeInsets.all(8.0),
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(
color: Colors.grey[200]!))),
child: TextFormField(
controller: groupIdController,
validator: (val) {
if (val!.isEmpty) {
return 'Please enter group id';
}
return null;
},
decoration: InputDecoration(
border: InputBorder.none,
hintText: "Group Id",
hintStyle: GoogleFonts.montserrat(
color: WidgetColors.HintColor,
fontSize: 14),
),
),
// ),
),
Container(
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(
color: Colors.grey[100]!))),
padding: const EdgeInsets.all(8.0),
child: TextFormField(
controller: userIdController,
validator: (val) {
if (val!.isEmpty) {
return 'Please enter user id';
}
return null;
},
decoration: InputDecoration(
border: InputBorder.none,
hintText:
"User Id", //"Please enter User ID",
// labelText: "User Id",
hintStyle: GoogleFonts.montserrat(
color: WidgetColors.HintColor,
fontSize: 14),
),
),
// ),
),
Container(
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(
color: Colors.grey[200]!))),
padding: const EdgeInsets.all(8.0),
child: TextFormField(
controller: passwordController,
obscureText: isVisible,
validator: (val) {
if (val!.isEmpty) {
return 'Please enter password';
}
return null;
},
decoration: InputDecoration(
border: InputBorder.none,
hintText: "Password",
hintStyle: GoogleFonts.montserrat(
color: WidgetColors.HintColor,
fontSize: 14),
suffixIcon: IconButton(
icon: isVisible
? const Icon(Icons.visibility_off)
: const Icon(Icons.visibility),
onPressed: () {
setState(() {
isVisible = !isVisible;
});
})),
),
//),
)
],
),
//)
),
//-Remember Me function UI Code
SizedBox(
height: 10,
),
Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
SizedBox(
height: 24.0,
width: 24.0,
child: Theme(
data: ThemeData(
unselectedWidgetColor:
Color(0xff00C8E8)),
child: Checkbox(
activeColor: Color(0xff00C8E8),
value: isCheckedRememberMe,
onChanged: actionRemeberMe(
isCheckedRememberMe)),
)),
SizedBox(width: 10.0),
Text("Remember Me",
style: TextStyle(
color: Color(0xff646464),
fontSize: 12,
))
]),
const SizedBox(
height: 30,
),
// FadeAnimation(
// 2,
Container(
height: 50,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(10),
gradient: const LinearGradient(colors: [
Color.fromRGBO(143, 148, 251, 10),
Color.fromRGBO(143, 148, 251, 20),
])),
child: StreamBuilder<Object>(
stream: bloc.isValid,
builder: (context, snapshot) {
return ElevatedButton(
style: ElevatedButton.styleFrom(
primary: WidgetColors.mainHeaderColor,
),
child: const Text(
'Submit',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold),
),
onPressed:
() //snapshot.hasError || !snapshot.hasData ? null :()
async {
},
),
InkWell(
onTap: () {},
child: Center(
child: Positioned(
child: Container(
margin: const EdgeInsets.only(top: 25),
child: const Center(
child: Text(
"Forgot Password ?",
style: TextStyle(
color: Colors.blue,
fontSize: 12,
fontWeight: FontWeight.normal),
),
),
//)
),
),
),
),
const SizedBox(
height: 70,
),
],
),
),
// ),
],
),
),
),
),
),
bottomNavigationBar: Container(
height: 30,
child: Row(
mainAxisAlignment: MainAxisAlignment.end,
// ignore: prefer_const_literals_to_create_immutables
children: [
Padding(
padding: const EdgeInsets.only(right: 10),
child:
const Text('4.2.18', style: TextStyle(color: Colors.black54)),
),
],
),
),
);
Conclusion
In this article, Implementing the Remember Me functionality in Flutter is quite simple when you understand how to use Local Storage. Being able to use this feature can make the user experience much smoother and help to ensure that users can quickly and reliably log back into an application without having to constantly enter their username and password. Additionally, it can help to improve the security of the application, allowing users to log in without having to constantly enter their credentials.
I hope it was a useful article, please share and subscribe to my channel, You have enjoyed the most. Thanks for reading and if you have any questions or comments, See you soon.