Hello developers, today I have to implement How to create a shipping address & add a user’s new delivery address
In flutter, the day-to-day creation of a new shopping/e-commerce app needs completion some challenge to create a developer for this type of app this topic help in your flutter app development directly and indirectly so I have to need form validation navigation and submit action with one screen to another screen jump in flutter app. So dear developers my aim is to provide very simple and point-to-point information on the related post with the help of flutter developers.
This topic covers –
- What is alert navigation from one to another screen in Flutter?
- How do I create a radio button for a ListView in flutter?
- How does Lsitview Bind with JSON Data in flutter?
- Listview.builder in flutter?
- Creating ListViews in flutter?
- Flutter ListView in doripot?
- Flutter ListView and ScrollPhysics in bind JSON.
- RadioButton inside ListView in flutter?
- Implementing Radio Buttons inside a ListView with flutter
- Dynamically create radio button Group in ListView.builder() flutter
- Select a specific radio button and get its value in ListView.builder Row click Flutter
- How to add a radio button in dynamically in a Flutter.
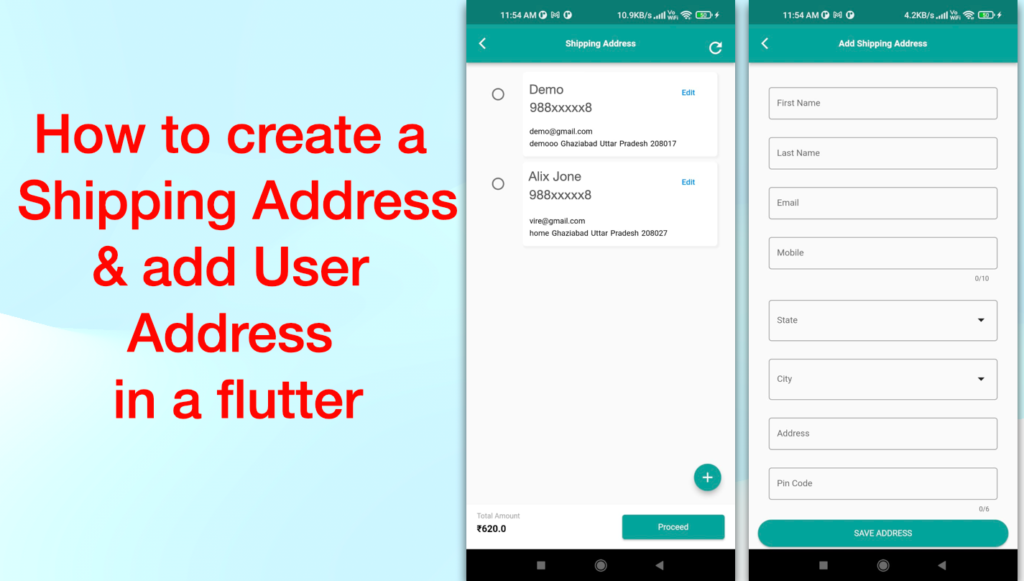
Shipping address list and add a new address screen a flutter.
Let’s Start to work
Address module in flutter (dart)
import 'dart:convert';
AddressMaster addressMasterFromJson(String str) =>
AddressMaster.fromJson(json.decode(str));
String addressMasterToJson(AddressMaster data) => json.encode(data.toJson());
class AddressMaster {
AddressMaster({
this.status,
this.message,
this.address,
});
String? status;
String? message;
List<Address>? address;
factory AddressMaster.fromJson(Map<String, dynamic> json) => AddressMaster(
status: json["status"],
message: json["message"],
address:
List<Address>.from(json["address"].map((x) => Address.fromJson(x))),
);
Map<String, dynamic> toJson() => {
"status": status,
"message": message,
"address": List<dynamic>.from(address!.map((x) => x.toJson())),
};
}
class Address {
Address({
this.addressId,
this.firstName,
this.lastName,
this.email,
this.phone,
this.address,
this.city,
this.cityName,
this.state,
this.stateName,
this.postalCode,
});
String? addressId;
String? firstName;
String? lastName;
String? email;
String? phone;
String? address;
String? city;
int? cityName;
String? state;
int? stateName;
String? postalCode;
factory Address.fromJson(Map<String, dynamic> json) => Address(
addressId: json["address_id"],
firstName: json["first_name"],
lastName: json["last_name"],
email: json["email"],
phone: json["phone"],
address: json["address"],
city: json["city"],
cityName: json["city_name"],
state: json["state"],
stateName: json["state_name"],
postalCode: json["postal_code"],
);
Map<String, dynamic> toJson() => {
"address_id": addressId,
"first_name": firstName,
"last_name": lastName,
"email": email,
"phone": phone,
"address": address,
"city": city,
"city_name": cityName,
"state": state,
"state_name": stateName,
"postal_code": postalCode,
};
}
Address Screen
I have used this screen in multiple languages formate.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: AppTheme.mainColor,
centerTitle: true,
leading: IconButton(
onPressed: () => Navigator.pop(context),
icon: const Icon(
Icons.arrow_back_ios,
size: 20,
color: Colors.white,
),
),
title: Text(
'MarkPlace_AddressTitles'.tr,// "Add Shipping Address",
style: CustomTextStyle.textFormFieldSemiBold.copyWith(
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
),
body: Container(
child: SingleChildScrollView(
padding: const EdgeInsets.symmetric(horizontal: 13, vertical: 10),
child: Form(
key: _formKey,
child: Column(
children: [
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 16, right: 16, top: 16),
child: TextFormField(
controller: firstNameController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'MarkPlace_FirstName'.tr,
hintText: 'MarkPlace_FirstName'.tr,
//icon: Icon(Icons.person),
isDense: true,
),
validator: (val) {
if (val!.isEmpty) {
return 'MarkPlace_FirstNameV'.tr;
}
return null;
},
),
),
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 16, right: 16, top: 16),
child: TextFormField(
controller: lastNameController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'MarkPlace_LastName'.tr,
hintText: 'MarkPlace_LastName'.tr,
//icon: Icon(Icons.person),
isDense: true,
),
validator: (val) {
if (val!.isEmpty) {
return 'MarkPlace_LastNameV'.tr;
}
return null;
},
),
),
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 16, right: 16, top: 16),
child: TextFormField(
controller: emailController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'MarkPlace_Email'.tr,
hintText: 'MarkPlace_Email'.tr,
//icon: Icon(Icons.person),
isDense: true,
),
),
),
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 16, right: 16, top: 16),
child: TextFormField(
controller: phoneController,
maxLength: 10,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'MarkPlace_ContactNo'.tr,
hintText: 'MarkPlace_ContactNo'.tr,
//icon: Icon(Icons.person),
isDense: true,
),
validator:
(val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = RegExp(patttern);
if (val!.isEmpty) {
return 'MarkPlace_mobileV'.tr;
} else if(val.length != 10){
return 'MarkPlace_MobileCV1'.tr;
}else if (!regExp.hasMatch(val)) {
return 'MarkPlace_MobileCV1'.tr;
}
return null;
},
inputFormatters: [
FilteringTextInputFormatter.allow(RegExp(r'[0.0-9.9]')),
],
),
),
const SizedBox(
height: 10,
),
Padding(
padding: EdgeInsets.only(left: 16, right: 16, top: 16),
child:
DropdownSearch<String>(
//value: _currentFarmerType,
mode: Mode.MENU,
showSelectedItems: true,
// showSelectedItem: true,
showSearchBox: true,
label: 'MarkPlace_State'.tr,
hint: 'MarkPlace_State'.tr,
popupItemDisabled: (String s) => s.startsWith('I'),
items: stateTypeI,
validator: (value) {
if (value == null) {
return 'MarkPlace_SateV'.tr;
}
},
onChanged: (val)
{
setState(() async {
_currentStateType = val!;
cityType.clear();
cityType = ['Please select city'];
_currentCityType = cityType[0];
getCitys();
});
getCitys();
}
),
),
const SizedBox(
height: 10,
),
Padding(
padding: EdgeInsets.only(left: 16, right: 16, top: 16),
child:
DropdownSearch<String>(
//value: _currentFarmerType,
mode: Mode.MENU,
showSelectedItems: true,
// showSelectedItem: true,
showSearchBox: true,
label: 'MarkPlace_City'.tr,
hint: 'MarkPlace_City'.tr,
popupItemDisabled: (String s) => s.startsWith('I'),
items: cityType,
validator: (value) {
if (value == null) {
return 'MarkPlace_CityV'.tr;
}
},
onChanged: (val) //=>
{
setState(() async {
_currentCityType = val!;
});
}
),
),
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 16, right: 16, top: 16),
child: TextFormField(
controller: addressDetailController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'MarkPlace_Address'.tr,
hintText: 'MarkPlace_Address'.tr,
//icon: Icon(Icons.person),
isDense: true,
),
validator: (val) {
if (val!.isEmpty) {
return 'MarkPlace_AddressV'.tr;
}
return null;
},
),
),
const SizedBox(
height: 10,
),
Padding(
padding: const EdgeInsets.only(left: 16, right: 16, top: 16),
child: TextFormField(
controller: pincodeController,
maxLength: 6,
// keyboardType: TextInputType.number,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'MarkPlace_PinCode'.tr,
hintText: 'MarkPlace_PinCode'.tr,
//icon: Icon(Icons.person),
isDense: true,
),
validator:
(val) {
String patttern = r'(^[0-9]*$)';
RegExp regExp = RegExp(patttern);
if (val!.isEmpty) {
return 'MarkPlace_PincodeV'.tr;
} else if(val.length != 6){
return 'MarkPlace_PinCV'.tr;
}
else if (!regExp.hasMatch(val)) {
return 'MarkPlace_PinCV1'.tr;
}
return null;
},
inputFormatters: [
FilteringTextInputFormatter.allow(RegExp(r'[0.0-9.9]')),
],
),
),
const SizedBox(
height: 10,
),
SizedBox(
width: double.infinity,
height: 40,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
primary: AppTheme.mainColor,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(40.0),
),
textStyle: CustomTextStyle.textFormFieldMedium.copyWith(
fontSize: 15,
fontWeight: FontWeight.normal,
),
),
onPressed: () async {
_submit();
},
child: Text('MarkPlace_BtnSaveAdd'.tr.toUpperCase()),
),
),
],
),
),
),
),
);
}
}
Conclusion:
In this article, we discussed the most beneficial part of the online shopping app adding shipping addresses with multiple addresses save on the database, Screen adds a floating action button to navigate an add new address screen with a button click, to make our development life easier In future parts, I will share some important code of How to create a Shipping Address in a flutter!, screen to make your Flutter Development journey a bit faster and many tips related to Flutter and Dart.
I hope it was a useful article, please share and subscribe to my channel, You have enjoyed the most. Thanks for reading and if you have any questions or comments, See you soon.